帮我编写爬虫程序,爬取电影网站(https://movie.douban.com/top250)文本数据,并保存至sqlite3数据库的源文件movies.db中;爬取网站全部图片数据,保存至项目的文件夹download中
时间: 2023-09-15 16:05:15 浏览: 212
### 回答1:
爬虫程序可以使用 Python 语言编写,下面是一个例子:
首先,我们需要安装相关的 Python 库,使用命令行工具或者 PyCharm 等 IDE 输入如下命令:
```
pip install requests BeautifulSoup4 lxml sqlite3
```
然后,我们可以使用 `requests` 库发送 HTTP 请求来获取网站的 HTML 源代码,使用 `BeautifulSoup4` 库解析 HTML 并获取相关的数据,使用 `lxml` 库作为 `BeautifulSoup4` 库的解析器。
```python
import requests
from bs4 import BeautifulSoup
url = "https://movie.douban.com/top250"
# 发送 HTTP 请求并获取响应内容
response = requests.get(url)
html = response.text
# 使用 BeautifulSoup 解析 HTML
soup = BeautifulSoup(html, "lxml")
```
接下来,我们可以使用 `soup.find_all()` 方法来查找 HTML 中的相关元素,并使用 `.text` 属性获取元素的文本内容,例如:
```python
# 获取所有电影的名称
names = []
for element in soup.find_all("span", class_="title"):
names.append(element.text)
print(names)
```
同样的,我们可以使用 `soup.find_all()` 方法来查找所有图片的 URL,并使用 `requests` 库的 `.get()` 方法获取图片数据,然后使用 `open()` 函数将图片数据写入文件:
```python
# 获取所有图片的 URL
img_urls = []
for element in soup.find_all("img"):
img_urls.append(element["src"])
# 下载图片
### 回答2:
编写爬虫程序可以使用Python编程语言,并借助第三方库BeautifulSoup和Requests。
首先,我们需要安装所需的库:
```
pip install beautifulsoup4
pip install requests
pip install sqlite3
```
接下来,编写Python爬虫程序:
```python
import requests
from bs4 import BeautifulSoup
import sqlite3
# 创建数据库连接
conn = sqlite3.connect('movies.db')
c = conn.cursor()
# 创建保存图片的文件夹
import os
if not os.path.exists('download'):
os.makedirs('download')
# 发送HTTP请求,获取网页内容
url = 'https://movie.douban.com/top250'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 解析电影信息
movies = soup.find_all('div', class_='item')
for movie in movies:
title = movie.find('span', class_='title').text
rating = movie.find('span', class_='rating_num').text
img_url = movie.find('img')['src']
# 存储电影数据至数据库
c.execute("INSERT INTO movies (title, rating) VALUES (?, ?)", (title, rating))
conn.commit()
# 存储图片至文件夹
img_data = requests.get(img_url).content
with open('download/' + title + '.jpg', 'wb') as f:
f.write(img_data)
# 关闭数据库连接
conn.close()
```
以上代码会首先创建一个名为movies.db的SQLite数据库文件,并在其中创建名为movies的表格。然后,它将爬取豆瓣电影Top250页面的电影信息和图片链接,并保存至数据库和项目文件夹中。
最后,运行该程序即可完成电影网站的文本数据和图片数据的爬取与保存。
### 回答3:
您好!为了编写爬虫程序来爬取电影网站https://movie.douban.com/top250 的文本数据,并保存至sqlite3数据库的源文件movies.db中,以及爬取网站全部图片数据并保存至项目的文件夹download中,您可以按照以下步骤进行:
1. 导入所需的库和模块:
```python
import requests
import sqlite3
import os
from bs4 import BeautifulSoup
```
2. 创建一个新的数据库并连接到数据库:
```python
conn = sqlite3.connect("movies.db")
cursor = conn.cursor()
cursor.execute("CREATE TABLE IF NOT EXISTS movies (id INTEGER PRIMARY KEY AUTOINCREMENT, title TEXT, rating REAL)")
```
3. 爬取网页数据,并解析文本数据和图片链接:
```python
url = "https://movie.douban.com/top250"
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
# 解析文本数据
movies = soup.find_all("div", class_="info")
for movie in movies:
title = movie.find("span", class_="title").get_text()
rating = movie.find("span", class_="rating_num").get_text()
cursor.execute("INSERT INTO movies (title, rating) VALUES (?, ?)", (title, rating))
# 解析图片链接
imgs = soup.find_all("img")
for img in imgs:
img_url = img["src"]
img_data = requests.get(img_url).content
file_name = os.path.basename(img_url)
with open(f"download/{file_name}", "wb") as f:
f.write(img_data)
```
4. 提交更改并关闭数据库连接:
```python
conn.commit()
conn.close()
```
以上代码将爬取电影网站的文本数据并保存至movies.db数据库中,同时也会将网站上的全部图片数据保存至项目的文件夹download中。
希望以上回答能对您有所帮助!如有其他问题,请随时提问。
阅读全文
相关推荐
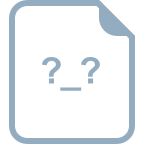
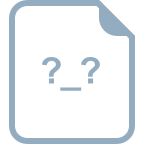

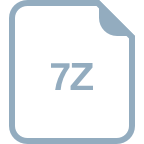
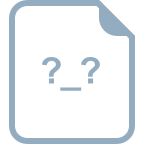
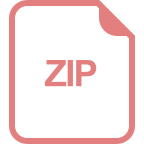
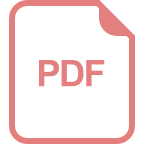
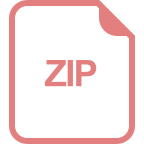
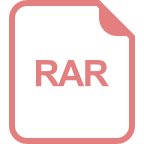
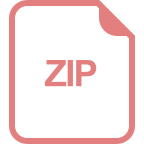
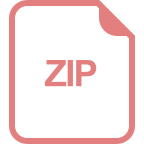
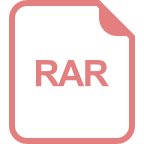
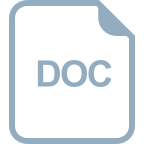
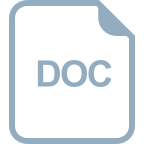
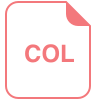


