编程算法用栈实现中缀表达式求值
时间: 2023-09-09 11:07:15 浏览: 92
1. 创建一个空栈。
2. 遍历表达式中的每个字符,如果是数字,则入栈。
3. 如果是运算符,则弹出栈顶的两个元素,进行运算,将结果入栈。
4. 重复步骤2和3,直到遍历完整个表达式。
5. 最后栈中只剩下一个元素,即为表达式的值。
具体实现如下:
```python
def evaluate(expression):
# 创建一个空栈
stack = []
# 定义运算符优先级
precedence = {'+': 1, '-': 1, '*': 2, '/': 2}
# 遍历表达式中的每个字符
for char in expression:
if char.isdigit():
# 如果是数字,则入栈
stack.append(int(char))
elif char in precedence:
# 如果是运算符,则弹出栈顶的两个元素,进行运算,将结果入栈
second_operand = stack.pop()
first_operand = stack.pop()
if char == '+':
result = first_operand + second_operand
elif char == '-':
result = first_operand - second_operand
elif char == '*':
result = first_operand * second_operand
else:
result = first_operand / second_operand
stack.append(result)
# 最后栈中只剩下一个元素,即为表达式的值
return stack.pop()
```
例如,对于表达式 "3+4*5-6/2",使用上述算法求解的结果为 20。
阅读全文
相关推荐





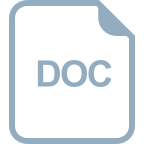









