使用c++编写输入一个中缀表达式,编程输出其后缀表达式,要求输出的后缀表达式的运算次序与输入的中缀表达式的运算次序相一致。
时间: 2024-03-26 19:35:30 浏览: 13
好的,这是一个算法问题,我可以帮你解答。
首先,我们可以使用栈来解决这个问题。遍历输入的中缀表达式,若遇到操作数则直接输出,若遇到运算符,则判断其与栈顶运算符的优先级,如果栈顶运算符优先级较高或相等,则将栈顶运算符弹出并输出,直到遇到优先级更低的运算符或左括号为止。最后将该运算符压入栈中。
具体的实现步骤如下:
1. 创建一个栈,用来存储运算符。
2. 遍历中缀表达式中的每个字符。
3. 如果是操作数,则直接输出。
4. 如果是左括号,则将其压入栈中。
5. 如果是右括号,则将栈中的运算符弹出并输出,直到遇到左括号为止。
6. 如果是运算符,则比较其与栈顶运算符的优先级,如果栈顶运算符优先级较高或相等,则将栈顶运算符弹出并输出,直到遇到优先级更低的运算符或左括号为止。最后将该运算符压入栈中。
7. 重复步骤2-6,直到遍历完整个中缀表达式。
8. 如果栈中还有运算符,则依次弹出并输出。
下面是C++代码实现(假设输入的中缀表达式已经存储在字符串中,且字符串中不含有空格):
```cpp
#include <iostream>
#include <stack>
#include <string>
using namespace std;
// 判断运算符优先级
int priority(char op) {
if (op == '+' || op == '-') {
return 1;
} else if (op == '*' || op == '/') {
return 2;
} else {
return 0;
}
}
// 中缀表达式转后缀表达式
string infixToPostfix(string infix) {
stack<char> s;
string postfix = "";
for (int i = 0; i < infix.length(); i++) {
char c = infix[i];
if (isdigit(c)) {
postfix += c;
} else if (c == '(') {
s.push(c);
} else if (c == ')') {
while (!s.empty() && s.top() != '(') {
postfix += s.top();
s.pop();
}
if (!s.empty()) {
s.pop(); // 弹出左括号
}
} else { // 运算符
while (!s.empty() && priority(c) <= priority(s.top())) {
postfix += s.top();
s.pop();
}
s.push(c);
}
}
while (!s.empty()) {
postfix += s.top();
s.pop();
}
return postfix;
}
int main() {
string infix;
cout << "请输入中缀表达式(不含空格):" << endl;
cin >> infix;
string postfix = infixToPostfix(infix);
cout << "后缀表达式为:" << postfix << endl;
return 0;
}
```
希望这个算法可以帮助你解决问题。如果还有其他问题,欢迎继续提问。
相关推荐
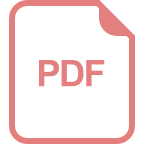














