用C++写一个中缀表达式转化后缀表达式的代码,要求以#结束输入并且任意表达式都可以运行
时间: 2024-05-16 12:12:56 浏览: 90
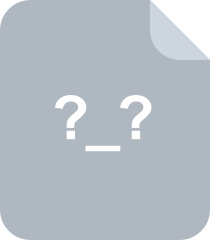
中缀表达式转后缀表达式的C++代码

以下是一个基于栈的中缀表达式转化后缀表达式的C++代码:
```C++
#include <iostream>
#include <stack>
#include <string>
using namespace std;
// 判断一个字符是否为运算符
bool isOperator(char c) {
return (c == '+' || c == '-' || c == '*' || c == '/');
}
// 判断运算符的优先级
int getPriority(char c) {
if (c == '*' || c == '/') {
return 2;
} else if (c == '+' || c == '-') {
return 1;
} else {
return 0;
}
}
// 中缀表达式转化为后缀表达式
string infixToPostfix(string infix) {
stack<char> s; // 运算符栈
string postfix; // 后缀表达式
for (int i = 0; i < infix.length(); i++) {
char c = infix[i];
if (c == ' ') { // 忽略空格
continue;
}
if (isdigit(c)) { // 是数字,直接加入后缀表达式
postfix += c;
} else if (isOperator(c)) { // 是运算符
while (!s.empty() && isOperator(s.top()) && getPriority(s.top()) >= getPriority(c)) {
// 栈顶是运算符且优先级比当前运算符高,弹出栈顶运算符加入后缀表达式
postfix += s.top();
s.pop();
}
// 当前运算符加入运算符栈
s.push(c);
} else if (c == '(') { // 是左括号,加入运算符栈
s.push(c);
} else if (c == ')') { // 是右括号,弹出运算符栈中左括号之前的所有运算符加入后缀表达式
while (!s.empty() && s.top() != '(') {
postfix += s.top();
s.pop();
}
if (!s.empty() && s.top() == '(') {
s.pop(); // 弹出左括号
}
}
}
// 将剩余的运算符加入后缀表达式
while (!s.empty()) {
postfix += s.top();
s.pop();
}
return postfix;
}
int main() {
string infix;
cout << "请输入中缀表达式,以#结束输入:" << endl;
getline(cin, infix, '#');
string postfix = infixToPostfix(infix);
cout << "后缀表达式为:" << postfix << endl;
return 0;
}
```
示例输入:
```
(1+2)*3-4/2#
```
示例输出:
```
后缀表达式为:12+3*42/-
```
阅读全文
相关推荐


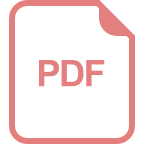









