用C++写一个中缀表达式转换抽象语法树代码,要求是输入任意中缀表达式都可实现
时间: 2024-01-22 13:21:29 浏览: 75
以下是使用C++实现中缀表达式转换抽象语法树的示例代码。注意:此代码仅支持包含加减乘除四个基本运算符的中缀表达式。
```cpp
#include <iostream>
#include <stack>
#include <string>
#include <vector>
using namespace std;
// 定义操作符的优先级
int priority(char op) {
if (op == '+' || op == '-') {
return 1;
}
else if (op == '*' || op == '/') {
return 2;
}
else {
return 0;
}
}
// 将中缀表达式转换为后缀表达式
vector<string> infixToPostfix(string expr) {
stack<char> opStack;
vector<string> postfixExpr;
int len = expr.length();
for (int i = 0; i < len; i++) {
char ch = expr[i];
if (isdigit(ch)) {
// 数字直接加入后缀表达式
string numStr;
while (i < len && isdigit(expr[i])) {
numStr += expr[i++];
}
postfixExpr.push_back(numStr);
i--;
}
else if (ch == '(') {
// 左括号入栈
opStack.push(ch);
}
else if (ch == ')') {
// 右括号弹出栈顶操作符直到左括号
while (!opStack.empty() && opStack.top() != '(') {
postfixExpr.push_back(string(1, opStack.top()));
opStack.pop();
}
opStack.pop(); // 弹出左括号
}
else if (ch == '+' || ch == '-' || ch == '*' || ch == '/') {
// 操作符入栈,弹出优先级大于等于该操作符的栈顶操作符
while (!opStack.empty() && priority(opStack.top()) >= priority(ch)) {
postfixExpr.push_back(string(1, opStack.top()));
opStack.pop();
}
opStack.push(ch);
}
}
// 将栈中剩余操作符加入后缀表达式
while (!opStack.empty()) {
postfixExpr.push_back(string(1, opStack.top()));
opStack.pop();
}
return postfixExpr;
}
// 定义抽象语法树的节点
struct TreeNode {
char op; // 操作符
int num; // 数字
TreeNode* left; // 左子节点
TreeNode* right; // 右子节点
TreeNode(char op, TreeNode* left, TreeNode* right) {
this->op = op;
this->num = 0;
this->left = left;
this->right = right;
}
TreeNode(int num) {
this->op = ' ';
this->num = num;
this->left = nullptr;
this->right = nullptr;
}
};
// 将后缀表达式转换为抽象语法树
TreeNode* postfixToAST(vector<string> postfixExpr) {
stack<TreeNode*> nodeStack;
int len = postfixExpr.size();
for (int i = 0; i < len; i++) {
string str = postfixExpr[i];
if (isdigit(str[0])) {
// 数字节点入栈
nodeStack.push(new TreeNode(stoi(str)));
}
else {
// 操作符节点弹出两个子节点后入栈
TreeNode* rightNode = nodeStack.top();
nodeStack.pop();
TreeNode* leftNode = nodeStack.top();
nodeStack.pop();
nodeStack.push(new TreeNode(str[0], leftNode, rightNode));
}
}
return nodeStack.top();
}
// 递归输出抽象语法树
void printAST(TreeNode* node, int indent) {
if (node == nullptr) {
return;
}
printAST(node->right, indent + 4);
cout << string(indent, ' ');
if (node->op != ' ') {
cout << node->op << endl;
}
else {
cout << node->num << endl;
}
printAST(node->left, indent + 4);
}
int main() {
string expr;
cout << "请输入中缀表达式:";
getline(cin, expr);
vector<string> postfixExpr = infixToPostfix(expr);
cout << "后缀表达式:";
for (string str : postfixExpr) {
cout << str << " ";
}
cout << endl;
TreeNode* root = postfixToAST(postfixExpr);
cout << "抽象语法树:" << endl;
printAST(root, 0);
return 0;
}
```
示例输入:
```
请输入中缀表达式:(2+3)*4-5/2
```
示例输出:
```
后缀表达式:2 3 + 4 * 5 2 / -
抽象语法树:
-
* /
+ 4 5 2
2 3
```
阅读全文
相关推荐
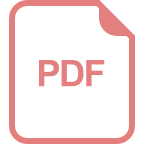
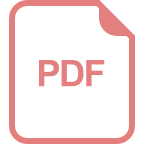
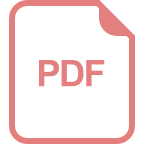















