中缀表达式转换成后缀c++代码
时间: 2024-10-07 09:02:11 浏览: 59
中缀表达式转后缀(也称逆波兰表示法,RPN)是一种将数学运算符放在操作数之后的表达式表示形式,常用于计算器和计算机内部处理。这里是一个简单的C++代码示例,使用栈数据结构来完成转换:
```cpp
#include <iostream>
#include <stack>
#include <string>
std::string infixToPostfix(const std::string& expression) {
// 定义操作符优先级
int priority[] = {0, 0, 1, 2, 2, 3}; // 0:空,1:* / %, 2: + - , 3: ()
std::stack<char> stack;
std::string postfix;
for (char c : expression) {
if (isdigit(c)) {
postfix += c; // 操作数直接添加到后缀
} else if (c == '(') {
stack.push(c);
} else if (c == ')') {
while (!stack.empty() && stack.top() != '(') {
postfix += stack.top();
stack.pop();
}
if (!stack.empty()) {
stack.pop(); // 移除左括号
}
} else {
while (!stack.empty() && priority[stack.top()] >= priority[c]) {
postfix += stack.top();
stack.pop();
}
stack.push(c); // 将当前操作符压入栈
}
}
// 处理剩余的操作符
while (!stack.empty()) {
postfix += stack.top();
stack.pop();
}
return postfix;
}
int main() {
std::string input = "A+B*C-D";
std::cout << "Infix: " << input << "\n";
std::string output = infixToPostfix(input);
std::cout << "Postfix: " << output << "\n";
return 0;
}
```
在这个例子中,我们遍历输入的中缀表达式,处理操作数、括号和运算符,并根据优先级规则逐步构建后缀表达式。当遇到右括号时,我们会先将其左侧的非括号元素全部弹出栈。
阅读全文
相关推荐
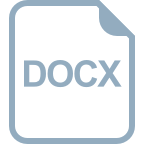
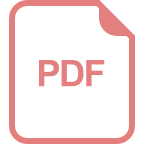
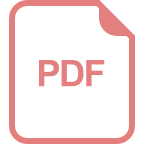















