中缀表达式转换成后缀表达式并求值的c++代码
时间: 2023-11-06 16:59:31 浏览: 100
以下是中缀表达式转换成后缀表达式并求值的C代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
#define STACK_SIZE 100
typedef struct {
int top;
int items[STACK_SIZE];
} Stack;
void push(Stack *s, int item) {
if (s->top >= STACK_SIZE) {
printf("Stack overflow\n");
exit(1);
}
s->items[++(s->top)] = item;
}
int pop(Stack *s) {
if (s->top <= -1) {
printf("Stack underflow\n");
exit(1);
}
return s->items[(s->top)--];
}
int priority(char operator) {
switch(operator) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
case '^':
return 3;
default:
return 0;
}
}
void infixToPostfix(char infix[], char postfix[]) {
Stack s;
s.top = -1;
int i, j;
for (i = 0, j = 0; infix[i] != '\0'; i++) {
if (isdigit(infix[i])) {
// operand
postfix[j++] = infix[i];
} else if (infix[i] == '(') {
// left parenthesis
push(&s, infix[i]);
} else if (infix[i] == ')') {
// right parenthesis
while (s.items[s.top] != '(') {
postfix[j++] = pop(&s);
}
pop(&s); // discard the left parenthesis
} else {
// operator
while (s.top != -1 && s.items[s.top] != '(' && priority(infix[i]) <= priority(s.items[s.top])) {
postfix[j++] = pop(&s);
}
push(&s, infix[i]);
}
}
// pop any remaining operators from the stack
while (s.top != -1) {
postfix[j++] = pop(&s);
}
postfix[j] = '\0'; // terminate the postfix string
}
int evaluatePostfix(char postfix[]) {
Stack s;
s.top = -1;
int i, op1, op2, result;
for (i = 0; postfix[i] != '\0'; i++) {
if (isdigit(postfix[i])) {
// operand
push(&s, postfix[i] - '0');
} else {
// operator
op2 = pop(&s);
op1 = pop(&s);
switch (postfix[i]) {
case '+':
result = op1 + op2;
break;
case '-':
result = op1 - op2;
break;
case '*':
result = op1 * op2;
break;
case '/':
result = op1 / op2;
break;
case '^':
result = 1;
for (int j = 0; j < op2; j++) {
result *= op1;
}
break;
}
push(&s, result);
}
}
return pop(&s);
}
int main() {
char infix[STACK_SIZE];
char postfix[STACK_SIZE];
printf("Enter an infix expression: ");
scanf("%s", infix);
infixToPostfix(infix, postfix);
printf("Postfix expression: %s\n", postfix);
printf("Result: %d\n", evaluatePostfix(postfix));
return 0;
}
```
此代码实现了中缀表达式转换成后缀表达式并求值的功能。其中,`infixToPostfix()`函数将中缀表达式转换成后缀表达式,`evaluatePostfix()`函数对后缀表达式求值,`priority()`函数返回操作符的优先级,`push()`和`pop()`函数分别实现栈的入栈和出栈操作。在`main()`函数中,用户输入一个中缀表达式,程序将其转换成后缀表达式并输出,然后求出后缀表达式的值并输出。
阅读全文
相关推荐






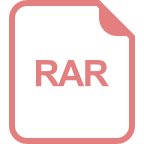








