中缀表达式转换为后缀表达式并求值用C++写出来的代码,且不用#include<stack>头文件和#include<vector>头文件
时间: 2023-06-14 13:04:56 浏览: 62
以下是使用数组模拟栈实现的代码:
```c++
#include<iostream>
#include<string>
#include<cstring>
#include<cstdlib>
using namespace std;
const int MAXN = 1000;
// 数组模拟栈
struct Stack {
int top;
int data[MAXN];
Stack() {
top = -1;
}
void push(int x) {
data[++top] = x;
}
int pop() {
return data[top--];
}
int peek() {
return data[top];
}
bool empty() {
return top == -1;
}
};
// 判断运算符优先级
int precedence(char op) {
if (op == '+' || op == '-') {
return 1;
} else if (op == '*' || op == '/') {
return 2;
} else if (op == '^') {
return 3;
} else {
return -1;
}
}
// 中缀表达式转后缀表达式
string infixToPostfix(string infix) {
string postfix;
Stack s;
for (int i = 0; i < infix.length(); i++) {
char c = infix[i];
if (isdigit(c)) {
postfix += c;
} else if (c == '(') {
s.push(c);
} else if (c == ')') {
while (!s.empty() && s.peek() != '(') {
postfix += s.pop();
}
s.pop();
} else {
while (!s.empty() && precedence(c) <= precedence(s.peek())) {
postfix += s.pop();
}
s.push(c);
}
}
while (!s.empty()) {
postfix += s.pop();
}
return postfix;
}
// 计算后缀表达式
int evaluatePostfix(string postfix) {
Stack s;
for (int i = 0; i < postfix.length(); i++) {
char c = postfix[i];
if (isdigit(c)) {
s.push(c - '0');
} else {
int b = s.pop();
int a = s.pop();
switch (c) {
case '+':
s.push(a + b);
break;
case '-':
s.push(a - b);
break;
case '*':
s.push(a * b);
break;
case '/':
s.push(a / b);
break;
case '^':
s.push(pow(a, b));
break;
}
}
}
return s.pop();
}
int main() {
string infix;
cout << "请输入中缀表达式:";
cin >> infix;
string postfix = infixToPostfix(infix);
cout << "后缀表达式为:" << postfix << endl;
int result = evaluatePostfix(postfix);
cout << "计算结果为:" << result << endl;
return 0;
}
```
注意:以上代码中使用了 `<cmath>` 头文件中的 `pow` 函数,如果不允许使用该头文件,可以自行实现幂运算函数。
相关推荐
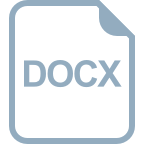
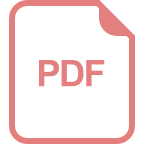
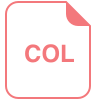













