编写代码中缀表达式转换为后缀表达式并求值
时间: 2023-10-09 18:05:53 浏览: 173
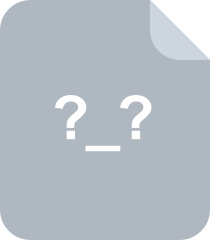
中缀表达式变换为后缀并用后缀表达式求值
好的,关于中缀表达式转换为后缀表达式的问题,可以使用栈来完成。具体方法是遍历中缀表达式,当遇到数字时直接输出(或者存储到结果栈中),当遇到运算符时需要将该运算符与栈顶运算符进行比较优先级,如果该运算符优先级较高,则直接压入栈中;如果栈顶运算符优先级较高或相等,则将栈顶运算符弹出并输出(或者存储到结果栈中),直到栈顶元素优先级小于该运算符,最后将该运算符压入栈中。完成中缀表达式的遍历后,如果栈中仍有元素,需要将它们全部弹出并输出(或者存储到结果栈中),即可得到后缀表达式。最后对后缀表达式进行求值,同样可以使用栈来完成。
阅读全文
相关推荐
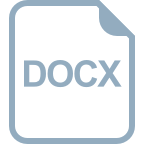
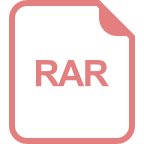
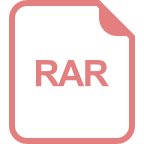
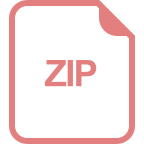
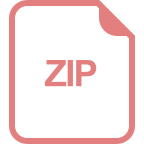
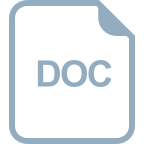


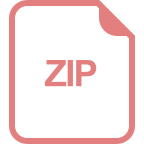
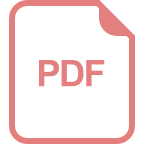
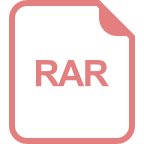





