中缀表达式转换为后缀表达式并求值
时间: 2023-10-09 17:07:27 浏览: 140
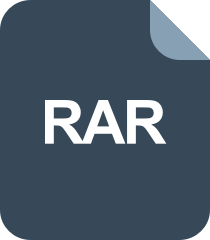
中缀表达式变后缀表达式的求值

首先需要将中缀表达式转换成后缀表达式。可以使用一个操作符栈来辅助转换过程。从左到右扫描中缀表达式的每个字符,并根据优先级进行相应的操作。
具体步骤如下:
1. 定义一个操作符栈和一个后缀表达式字符串。
2. 从左到右扫描中缀表达式的每个字符:
- 如果遇到数字,直接添加到后缀表达式字符串中。
- 如果遇到左括号,将其压入操作符栈。
- 如果遇到右括号,弹出操作符栈中的操作符,并将其添加到后缀表达式字符串中,直到遇到左括号为止,并将左括号从操作符栈中弹出。
- 如果遇到操作符,比较其与操作符栈栈顶操作符的优先级:
- 如果该操作符优先级大于栈顶操作符优先级,将其压入操作符栈。
- 如果该操作符优先级小于等于栈顶操作符优先级,将栈顶操作符弹出,并将其添加到后缀表达式字符串中,直到栈顶操作符优先级小于该操作符,或者栈为空,然后将该操作符压入操作符栈。
3. 扫描完中缀表达式后,将操作符栈中的所有操作符依次弹出,并添加到后缀表达式字符串中。
4. 最后,后缀表达式字符串即为转换后的后缀表达式。
接下来是对后缀表达式进行求值的过程:
1. 定义一个数值栈。
2. 从左到右扫描后缀表达式的每个字符:
- 如果遇到数字,将其转换成数值并压入数值栈。
- 如果遇到操作符,从数值栈中弹出两个数值,进行相应的操作,并将结果压入数值栈。
3. 扫描完后缀表达式后,数值栈中的唯一元素即为求值结果。
综上所述,将中缀表达式转换为后缀表达式并求值的过程如上所述。
阅读全文
相关推荐
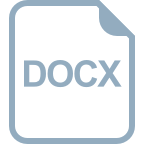




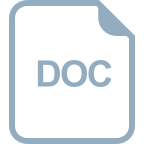








