写一个栈将中缀表达式转换为后缀表达式并求值的代码
时间: 2023-10-09 19:04:40 浏览: 100
好的,以下是代码实现:
```python
# 定义运算符优先级
priority_dict = {'(': 0, '+': 1, '-': 1, '*': 2, '/': 2, '%': 2, '^': 3}
# 中缀表达式转换为后缀表达式并求值
def infix_to_postfix(expression):
postfix_list = []
operator_stack = []
for i in expression:
if i.isdigit():
postfix_list.append(i)
elif i == '(':
operator_stack.append(i)
elif i == ')':
while operator_stack[-1] != '(':
postfix_list.append(operator_stack.pop())
operator_stack.pop()
else:
while operator_stack and priority_dict[i] <= priority_dict[operator_stack[-1]]:
postfix_list.append(operator_stack.pop())
operator_stack.append(i)
while operator_stack:
postfix_list.append(operator_stack.pop())
postfix_expression = ''.join(postfix_list)
return postfix_expression
# 计算后缀表达式
def postfix_eval(expression):
operand_stack = []
for i in expression:
if i.isdigit():
operand_stack.append(int(i))
else:
operand2 = operand_stack.pop()
operand1 = operand_stack.pop()
result = 0
if i == '+':
result = operand1 + operand2
elif i == '-':
result = operand1 - operand2
elif i == '*':
result = operand1 * operand2
elif i == '/':
result = operand1 / operand2
elif i == '%':
result = operand1 % operand2
elif i == '^':
result = operand1 ** operand2
operand_stack.append(result)
return operand_stack.pop()
# 测试代码
expression = input('请输入中缀表达式:')
postfix_expression = infix_to_postfix(expression)
print('中缀表达式转换为后缀表达式:', postfix_expression)
result = postfix_eval(postfix_expression)
print('计算结果为:', result)
```
请注意,该代码只能处理数字和加减乘除取模幂等基本运算符,不能处理括号外的运算符优先级问题。如果表达式中包含非法字符或表达式格式不正确,代码将会报错。
阅读全文
相关推荐

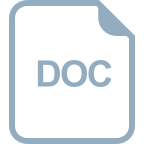













