用C++写一个中缀表达式转化后缀表达式的代码,要求以#结束输入并且任意表达式都可以运行
时间: 2024-05-10 19:17:10 浏览: 7
以下是用C++实现中缀表达式转化为后缀表达式的代码:
```c++
#include <iostream>
#include <stack>
#include <string>
using namespace std;
// 定义运算符优先级函数
int priority(char op) {
switch (op) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
case '^':
return 3;
default:
return 0;
}
}
// 中缀表达式转化为后缀表达式函数
string infixToPostfix(string infix) {
stack<char> s;
string postfix;
int len = infix.size();
for (int i = 0; i < len; i++) {
char c = infix[i];
if (isdigit(c) || isalpha(c)) { // 如果是数字或字母,直接输出
postfix += c;
} else if (c == '(') { // 如果是左括号,入栈
s.push(c);
} else if (c == ')') { // 如果是右括号,弹出栈中左括号之前的所有运算符并输出
while (!s.empty() && s.top() != '(') {
postfix += s.top();
s.pop();
}
if (!s.empty()) { // 弹出左括号
s.pop();
}
} else { // 如果是运算符,弹出栈中优先级大于等于该运算符的运算符并输出,然后将该运算符入栈
while (!s.empty() && priority(s.top()) >= priority(c)) {
postfix += s.top();
s.pop();
}
s.push(c);
}
}
// 将栈中剩余的运算符全部输出
while (!s.empty()) {
postfix += s.top();
s.pop();
}
return postfix;
}
int main() {
string infix;
cout << "请输入中缀表达式(以#结束):" << endl;
cin >> infix;
string postfix = infixToPostfix(infix);
cout << "后缀表达式为:" << postfix << endl;
return 0;
}
```
该程序可以处理包含括号、加减乘除、幂运算以及任意数目的数字和变量的中缀表达式,并将其转化为后缀表达式。
相关推荐
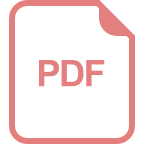














