用C++写一个输入任意中缀表达式转换抽象语法树代码
时间: 2023-12-11 10:05:34 浏览: 89
以下是使用C++实现输入任意中缀表达式转换抽象语法树的示例代码:
```c++
#include <iostream>
#include <stack>
#include <string>
#include <sstream>
using namespace std;
struct TreeNode {
string val;
TreeNode* left;
TreeNode* right;
TreeNode(string v): val(v), left(NULL), right(NULL) {}
};
bool isOperator(string s) {
return s == "+" || s == "-" || s == "*" || s == "/";
}
int getPriority(string op) {
if (op == "+" || op == "-") {
return 1;
} else if (op == "*" || op == "/") {
return 2;
} else {
return 0;
}
}
TreeNode* buildAST(string expression) {
stack<TreeNode*> nodeStack;
stack<string> opStack;
stringstream ss(expression);
string token;
while (ss >> token) {
if (isOperator(token)) {
while (!opStack.empty() && getPriority(opStack.top()) >= getPriority(token)) {
TreeNode* right = nodeStack.top();
nodeStack.pop();
TreeNode* left = nodeStack.top();
nodeStack.pop();
string op = opStack.top();
opStack.pop();
TreeNode* newNode = new TreeNode(op);
newNode->left = left;
newNode->right = right;
nodeStack.push(newNode);
}
opStack.push(token);
} else {
TreeNode* newNode = new TreeNode(token);
nodeStack.push(newNode);
}
}
while (!opStack.empty()) {
TreeNode* right = nodeStack.top();
nodeStack.pop();
TreeNode* left = nodeStack.top();
nodeStack.pop();
string op = opStack.top();
opStack.pop();
TreeNode* newNode = new TreeNode(op);
newNode->left = left;
newNode->right = right;
nodeStack.push(newNode);
}
return nodeStack.top();
}
void inorder(TreeNode* root) {
if (root == NULL) return;
if (root->left != NULL) {
cout << "(";
inorder(root->left);
}
cout << root->val;
if (root->right != NULL) {
inorder(root->right);
cout << ")";
}
}
int main() {
string expression = "3 + 4 * 5 - 6 / 2";
TreeNode* root = buildAST(expression);
inorder(root);
cout << endl;
return 0;
}
```
该示例代码实现了输入任意中缀表达式,将其转换为抽象语法树,并输出其对应的中缀表达式形式。其中,`buildAST`函数实现了将中缀表达式转换为抽象语法树的过程,`inorder`函数实现了中序遍历抽象语法树并输出中缀表达式的过程。
阅读全文
相关推荐
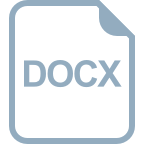
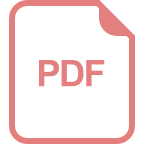
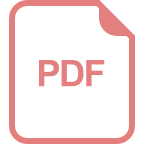















