用c++写一个由中缀表达式变为后缀表达式的函数
时间: 2024-10-03 11:04:51 浏览: 61
在C++中,将中缀表达式转换成后缀表达式,也称为逆波兰表示法(Reverse Polish Notation,RPN),可以使用栈的数据结构。这里提供一个简单的递归解析方法示例:
```cpp
#include <iostream>
#include <stack>
#include <string>
// 定义操作符优先级
int precedence(char op) {
if (op == '+' || op == '-') return 1;
if (op == '*' || op == '/') return 2;
return 0;
}
// 将字符添加到结果后缀表达式
void appendToRPN(std::string &rpn, std::stack<char> &ops) {
rpn += ops.top();
ops.pop();
}
// 中缀表达式转后缀表达式的主函数
std::string infixToPostfix(const std::string &expr) {
std::stack<char> ops; // 栈用于存放操作符
std::string rpn = ""; // 后缀表达式
for (char c : expr) {
// 检查字符是否是数字或操作数,如果是就直接加入RPN
if (isdigit(c)) {
rpn += c;
} else if (c == '(') { // 左括号入栈
ops.push(c);
} else if (c == ')') { // 右括号出栈并添加所有操作符
while (!ops.empty() && ops.top() != '(') {
appendToRPN(rpn, ops);
}
ops.pop(); // 出栈左括号
} else { // 操作符处理
while (!ops.empty() && precedence(c) <= precedence(ops.top())) {
appendToRPN(rpn, ops);
}
ops.push(c); // 把当前操作符压入栈中
}
}
// 处理剩余的操作符
while (!ops.empty()) {
appendToRPN(rpn, ops);
}
return rpn;
}
// 测试函数
int main() {
std::string expr = "A + B * C / D"; // 示例中缀表达式
std::cout << "Infix: " << expr << "\n";
std::cout << "Postfix: " << infixToPostfix(expr) << "\n";
return 0;
}
```
在这个例子中,`infixToPostfix`函数会遍历输入的中缀表达式,根据操作符的优先级和括号来构造后缀表达式。请注意,这个函数假设了输入的中缀表达式是有效的。
阅读全文
相关推荐
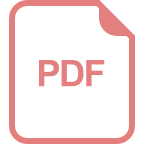
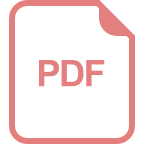
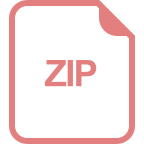


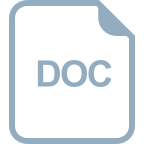
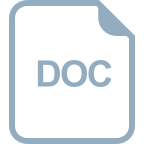
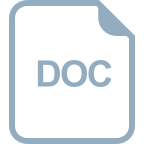
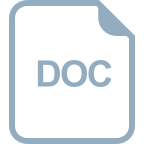
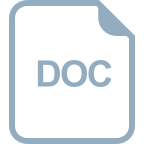
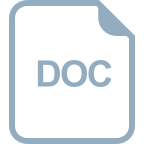







