写一个根据后缀表达式输出中缀表达式的函数c++
时间: 2024-12-18 14:32:14 浏览: 6
在C++中,我们可以使用栈数据结构来转换后缀表达式(也称为逆波兰表示法,RPN)到中缀表达式。以下是一个简单的函数,它接受一个后缀表达式的字符串作为输入并返回对应的中缀表达式:
```cpp
#include <string>
#include <stack>
#include <map>
// 定义操作符优先级,可以根据实际需求调整
std::map<char, int> precedence = { {'*', 2}, {'/', 2}, {'+', 1}, {'-', 1} };
std::string infixFromPostfix(const std::string& postfixExp) {
std::stack<char> opStack;
std::string output;
for (char c : postfixExp) {
if (isdigit(c)) { // 数字直接加入结果
output += c;
} else if (isalpha(c)) { // 处理变量
output += c;
} else if (c == '(') { // 左括号入栈
opStack.push(c);
} else if (c == ')') { // 右括号出栈,直到遇到左括号
while (!opStack.empty() && opStack.top() != '(') {
output += opStack.top();
opStack.pop();
}
if (!opStack.empty()) {
opStack.pop(); // 出栈左括号
}
} else { // 操作符
while (!opStack.empty() && precedence[c] <= precedence[opStack.top()]) {
output += opStack.top();
opStack.pop();
}
opStack.push(c);
}
}
// 清理剩余的操作符
while (!opStack.empty()) {
output += opStack.top();
opStack.pop();
}
return output;
}
int main() {
std::string postfixExp = "3+4*5*(2-1)";
std::string infixExp = infixFromPostfix(postfixExp);
std::cout << "Infix expression: " << infixExp << std::endl;
return 0;
}
```
这个函数首先遍历后缀表达式的每一个字符,如果遇到数字则直接追加到结果,如果是变量字符或左括号则不变;遇到右括号时,会将栈顶的所有操作符和左括号依次弹出;遇到操作符时,比较其优先级,只有当栈顶操作符的优先级小于等于当前操作符时才会将其压回结果。最后,清理剩余的操作符。
阅读全文
相关推荐
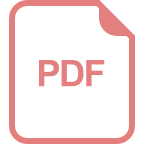
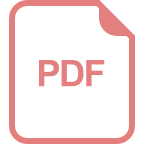
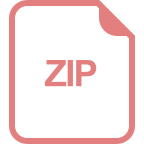















