实现简易计算器,包括后缀表达式计算、中缀表达式计算和中缀转后缀表达式。其中,操作符包括加、减、乘、除,操作数为0-9之间的整数。利用c++语言实现,并输出代码
时间: 2024-10-19 17:12:13 浏览: 46
实现一个简单的计算器可以分为几个步骤:后缀表达式(也称为逆波兰表示法,RPN)的计算、中缀表达式的计算以及中缀表达式到后缀表达式的转换。以下是使用C++基本语法的示例代码:
**1. 后缀表达式计算:**
```cpp
#include <iostream>
#include <stack>
using namespace std;
int precedence(char op) {
if (op == '+' || op == '-') return 1;
else if (op == '*' || op == '/') return 2;
return 0;
}
int applyOp(int a, int b, char op) {
switch (op) {
case '+': return a + b;
case '-': return a - b;
case '*': return a * b;
case '/': return a / b;
}
}
int evaluatePostfix(string postFix) {
stack<int> s;
for (char c : postFix) {
if (isdigit(c)) {
s.push(c - '0');
} else {
int b = s.top(); s.pop();
int a = s.top(); s.pop();
s.push(applyOp(a, b, c));
}
}
return s.top();
}
int main() {
string postfixExp = "2 3 + 4 *";
cout << "Result of RPN expression: " << evaluatePostfix(postfixExp) << endl;
return 0;
}
```
**2. 中缀表达式计算:**
```cpp
#include <stack>
#include <string>
bool isOperator(char c) {
return c == '+' || c == '-' || c == '*' || c == '/';
}
int calculateInfix(string infixExp) {
stack<char> ops;
for (size_t i = 0; i < infixExp.length(); ++i) {
if (isOperator(infixExp[i])) {
while (!ops.empty() && precedence(ops.top()) >= precedence(infixExp[i])) {
int b = popOperand();
int a = popOperand();
performOperation(a, b, ops.top());
pushOperand(result);
ops.pop();
}
ops.push(infixExp[i]);
} else {
pushOperand(stoi(infixExp.substr(i, 1)));
}
}
while (!ops.empty()) {
int b = popOperand();
int a = popOperand();
performOperation(a, b, ops.top());
pushOperand(result);
ops.pop();
}
return popOperand();
}
// 其他辅助函数省略...
```
**3. 中缀转后缀表达式:Shunting Yard算法**
这是一个更复杂的过程,通常涉及构建一个队列和两个栈。这里只提供简化版伪代码:
```cpp
void infixToPostfix(string &infix) {
// ... 使用栈和队列执行Shunting Yard算法 ...
}
```
完整的中缀转后缀的代码会涉及到递归和更多的细节,不是一段简短的代码能完全展示。
以上代码片段展示了关键部分,完整实现需要连接各个部分并处理异常情况。运行`calculateInfix()`函数之前,你需要先将输入的中缀表达式转成后缀表达式,然后再计算。
阅读全文
相关推荐
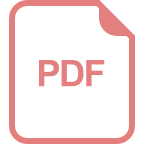
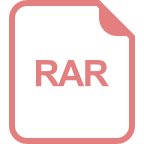
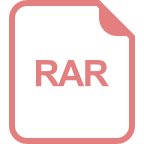



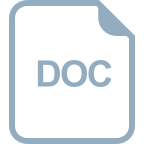
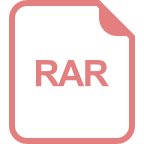
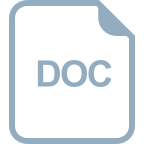
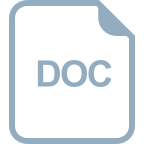
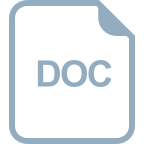
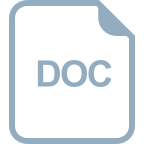
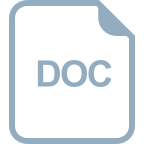

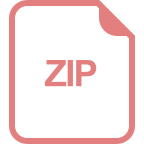
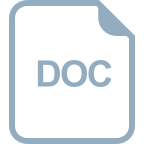
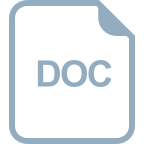
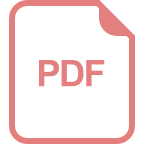
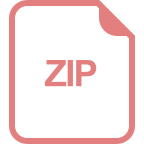