使用c++,生成实现简易计算器,包括后缀表达式计算、中缀表达式计算和中缀转后缀表达式。其中,操作符包括加、减、乘、除,操作数为0-9之间的整数的代码。
时间: 2024-10-23 07:11:22 浏览: 33
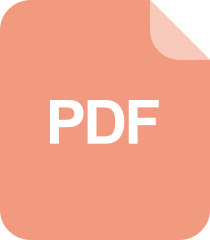
C++实现中缀表达式转后缀表达式
在C++中实现一个简易计算器,可以分为三个部分:后缀表达式(Reverse Polish Notation,RPN)计算、中缀表达式计算以及中缀表达式转后缀表达式。这里我会给出一个简化版本的代码示例,注意这只是一个基础实现,并未包含错误处理和复杂的优化。
**1. 后缀表达式计算(RPN):**
```cpp
#include <iostream>
#include <stack>
using namespace std;
int evalPostfix(string tokens) {
stack<int> s;
for (char token : tokens) {
if (isdigit(token)) {
s.push(stoi(token));
} else {
int second = s.top(); s.pop();
int first = s.top(); s.pop();
switch (token) {
case '+': s.push(first + second); break;
case '-': s.push(first - second); break;
case '*': s.push(first * second); break;
case '/': s.push(first / second); break;
default: assert(false);
}
}
}
return s.top();
}
string calculatePostfix(string infix) {
// ... 实现中缀到后缀的转换,这里省略
return postfix;
}
int main() {
string expression = "2 3 + 4 *";
cout << "Result of RPN: " << evalPostfix(calculatePostfix(expression)) << endl;
return 0;
}
```
**2. 中缀表达式计算:**
```cpp
bool isOperator(char c) { return c == '+' || c == '-' || c == '*' || c == '/'; }
int evalInfix(string infix) {
stack<pair<char, int>> ops; // Storing operators and their priority
int num = 0;
for (char c : infix) {
if (isdigit(c)) {
num = num * 10 + c - '0';
} else if (isOperator(c)) {
while (!ops.empty() && hasHigherPrecedence(ops.top().first, c)) {
// Perform operation
char op = ops.top().first;
int right = num;
num = ops.top().second;
ops.pop();
num = applyOperation(num, right, op);
}
ops.push({c, num});
} else {
assert(false);
}
}
while (!ops.empty()) {
char op = ops.top().first;
int right = num;
num = ops.top().second;
ops.pop();
num = applyOperation(num, right, op);
}
return num;
}
// Apply the operator to two operands
int applyOperation(int left, int right, char op) {
switch (op) {
case '+': return left + right;
case '-': return left - right;
case '*': return left * right;
case '/': return left / right;
default: assert(false);
}
}
int main() {
string expression = "2 + 3 * 4";
cout << "Result of infix: " << evalInfix(expression) << endl;
return 0;
}
```
**3. 中缀转后缀表达式:**
这部分通常涉及先序遍历(Preorder Traversal)和栈操作,这里仅提供思路:
```cpp
void infixToPostfix(string& infix) {
stack<char> ops;
// ... 实现算法,这里省略
}
string infixExpressionToPostfix(string input) {
// Split input by spaces or symbols, then process each part
return infixToPostfix(input);
}
```
**
阅读全文
相关推荐
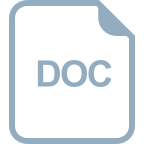
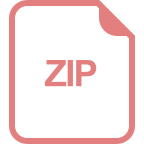









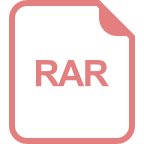
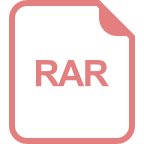
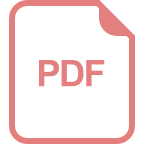
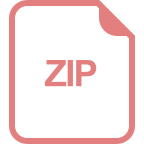
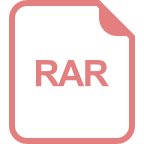
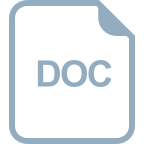
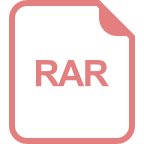