完善一个计算器类型定义,实现中缀表达式转换成后缀表达式、中缀表达式的计算(相当于后缀表达式的解析)
时间: 2024-06-17 08:03:19 浏览: 262
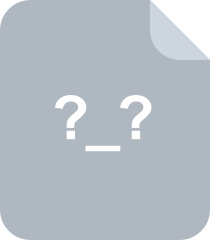
中缀表达式计算器实现

一个计算器类型定义通常需要包含以下几个部分:
1. 数据结构部分:用于存储操作数和运算符的栈,以及存储后缀表达式的队列。
2. 中缀表达式转后缀表达式的算法实现:将中缀表达式依次扫描,将操作数直接加入后缀表达式队列中,将运算符压入栈中,并按照优先级进行比较,如果栈顶运算符优先级大于等于当前运算符,则将栈顶运算符弹出并加入后缀表达式队列中,直到栈顶运算符优先级小于当前运算符或者栈为空,然后将当前运算符入栈。扫描完中缀表达式后,将栈中剩余的运算符依次弹出并加入后缀表达式队列中。
3. 后缀表达式计算的算法实现:依次扫描后缀表达式,遇到操作数则压入栈中,遇到运算符则从栈中弹出相应数量的操作数,进行运算,并将结果压入栈中。最终栈中剩下的唯一一个元素就是计算结果。
以下是一个示例代码片段:
```
#include <iostream>
#include <stack>
#include <queue>
#include <string>
using namespace std;
int main() {
stack<char> opStack; // 操作符栈
queue<string> postfixQueue; // 后缀表达式队列
string infix; // 中缀表达式
cin >> infix;
int len = infix.length();
for (int i = 0; i < len; i++) {
char c = infix[i];
if (isdigit(c)) {
int j = i + 1;
while (j < len && isdigit(infix[j])) j++;
postfixQueue.push(infix.substr(i, j - i));
i = j - 1;
} else if (c == '(') {
opStack.push(c);
} else if (c == ')') {
while (opStack.top() != '(') {
postfixQueue.push(string(1, opStack.top()));
opStack.pop();
}
opStack.pop(); // 弹出左括号
} else {
while (!opStack.empty() && opStack.top() != '(' && ((c == '*' || c == '/') ? (opStack.top() == '*' || opStack.top() == '/') : true)) {
postfixQueue.push(string(1, opStack.top()));
opStack.pop();
}
opStack.push(c);
}
}
while (!opStack.empty()) {
postfixQueue.push(string(1, opStack.top()));
opStack.pop();
}
stack<double> numStack; // 操作数栈
while (!postfixQueue.empty()) {
string token = postfixQueue.front();
postfixQueue.pop();
if (isdigit(token)) {
numStack.push(stod(token));
} else {
double b = numStack.top();
numStack.pop();
double a = numStack.top();
numStack.pop();
switch (token) {
case '+': numStack.push(a + b); break;
case '-': numStack.push(a - b); break;
case '*': numStack.push(a * b); break;
case '/': numStack.push(a / b); break;
default: break;
}
}
}
cout << numStack.top() << endl;
return 0;
}
```
阅读全文
相关推荐
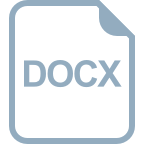
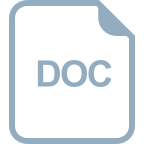
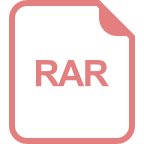
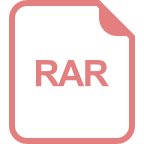
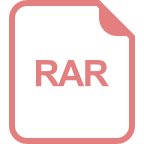
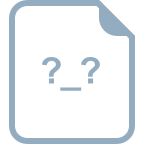
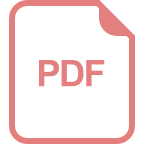
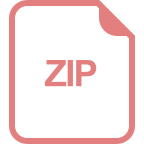
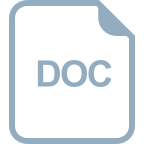
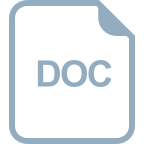
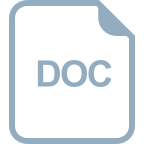
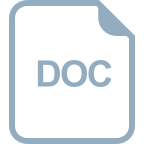
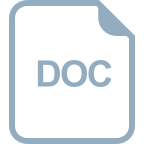


