中缀表达式转换成后缀表达式,生成一个完整的c语言代码
时间: 2024-10-16 21:04:52 浏览: 98
中缀表达式(也称为前缀表达式)转换为后缀表达式(也称为逆波兰表示法或RPN),是一种常见的算法问题,常用于计算器的设计等场景。以下是将中缀表达式转换为后缀表达式的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义操作数栈
typedef struct Stack {
int *arr;
int top;
} Stack;
void push(Stack *stack, int val) {
if (stack->top == -1) {
stack->arr = (int*)malloc(sizeof(int));
stack->top = 0;
}
stack->arr[++stack->top] = val;
}
int pop(Stack *stack) {
return stack->arr[stack->top--];
}
char* infixToPostfix(char* infix) {
char* postfix = NULL; // 后缀表达式结果
int prec[] = {'+':1, '-':1, '*':2, '/':2}; // 运算符优先级
Stack s;
s.top = -1;
// 遍历输入字符串
for (int i = 0; infix[i]; ++i) {
if (isdigit(infix[i])) { // 数字直接入栈
postfix = (char*)realloc(postfix, (strlen(postfix) + 2) * sizeof(char));
postfix[strlen(postfix)] = infix[i];
postfix[strlen(postfix) + 1] = '\0';
} else if (infix[i] == '(') { // 左括号入栈
push(&s, i);
} else if (infix[i] == ')') { // 右括号出栈并处理运算
while (s.top != -1 && s.arr[s.top] != '(') {
postfix = (char*)realloc(postfix, (strlen(postfix) + 3) * sizeof(char));
postfix[strlen(postfix)] = ')';
postfix[strlen(postfix) + 1] = s.arr[s.top];
postfix[strlen(postfix) + 2] = ' ';
s.top--;
postfix[strlen(postfix)] = infix[i];
postfix[strlen(postfix) + 1] = '\0';
}
s.top--;
} else { // 操作符按优先级处理
while (s.top != -1 && prec[infix[i]] <= prec[s.arr[s.top]]) {
postfix = (char*)realloc(postfix, (strlen(postfix) + 3) * sizeof(char));
postfix[strlen(postfix)] = ')';
postfix[strlen(postfix) + 1] = s.arr[s.top];
postfix[strlen(postfix) + 2] = ' ';
s.top--;
}
push(&s, i); // 入栈操作符
}
}
// 处理剩余的左括号和操作数
while (s.top != -1) {
postfix = (char*)realloc(postfix, (strlen(postfix) + 3) * sizeof(char));
postfix[strlen(postfix)] = ')';
postfix[strlen(postfix) + 1] = s.arr[s.top];
postfix[strlen(postfix) + 2] = ' ';
s.top--;
}
postfix[strlen(postfix)] = '\0'; // 结束符号
return postfix;
}
int main() {
char infix[] = "A+B*C-(D+E)";
printf("Infix: %s\n", infix);
char* postfix = infixToPostfix(infix);
printf("Postfix: %s\n", postfix);
free(postfix);
return 0;
}
```
这段代码定义了一个`infixToPostfix`函数,它接受一个中缀表达式作为输入,并返回相应的后缀表达式。在`main`函数中,我们测试了一个例子。
阅读全文
相关推荐
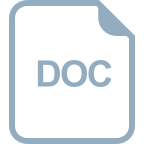
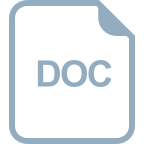
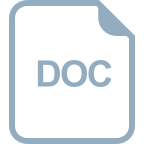
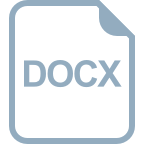
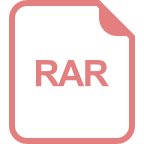

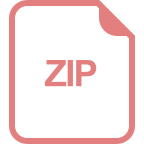
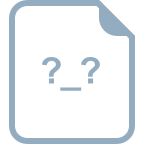
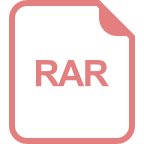
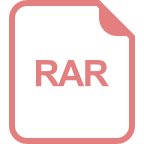







