输入字符实现中缀式转换后缀式,,词法分析,语法分析,中间代码生成,输出代码实现
时间: 2024-03-06 19:50:28 浏览: 29
好的,我来为你提供一个简单的代码实现。下面的代码实现使用 Python 语言,包括中缀式转换后缀式、词法分析、语法分析和中间代码生成,输出部分可以根据具体情况进行修改。
```python
# 中缀式转换后缀式
def infix_to_postfix(expression):
priority = {'+': 1, '-': 1, '*': 2, '/': 2, '(': 0}
stack = []
postfix_expression = []
for token in expression:
if token.isdigit():
postfix_expression.append(token)
elif token in '+-*/':
while stack and priority[token] <= priority[stack[-1]]:
postfix_expression.append(stack.pop())
stack.append(token)
elif token == '(':
stack.append(token)
elif token == ')':
while stack[-1] != '(':
postfix_expression.append(stack.pop())
stack.pop()
while stack:
postfix_expression.append(stack.pop())
return postfix_expression
# 词法分析
def lexical_analysis(expression):
tokens = []
current_token = ''
for token in expression:
if token.isdigit() or token.isalpha():
current_token += token
else:
if current_token:
tokens.append(current_token)
current_token = ''
if token != ' ':
tokens.append(token)
if current_token:
tokens.append(current_token)
return tokens
# 语法分析
def parse_expression(tokens):
stack = []
for token in tokens:
if token.isdigit():
stack.append(('push', token))
elif token == '+':
right = stack.pop()
left = stack.pop()
stack.append(('add', left, right))
elif token == '-':
right = stack.pop()
left = stack.pop()
stack.append(('sub', left, right))
elif token == '*':
right = stack.pop()
left = stack.pop()
stack.append(('mul', left, right))
elif token == '/':
right = stack.pop()
left = stack.pop()
stack.append(('div', left, right))
return stack[0]
# 中间代码生成
def generate_code(expression):
postfix_expression = infix_to_postfix(expression)
tokens = lexical_analysis(postfix_expression)
parse_tree = parse_expression(tokens)
code = []
for instruction in parse_tree:
if instruction[0] == 'push':
code.append(f'push {instruction[1]}')
elif instruction[0] == 'add':
code.append('pop ebx')
code.append('pop eax')
code.append('add eax, ebx')
code.append('push eax')
elif instruction[0] == 'sub':
code.append('pop ebx')
code.append('pop eax')
code.append('sub eax, ebx')
code.append('push eax')
elif instruction[0] == 'mul':
code.append('pop ebx')
code.append('pop eax')
code.append('imul eax, ebx')
code.append('push eax')
elif instruction[0] == 'div':
code.append('pop ebx')
code.append('pop eax')
code.append('cdq')
code.append('idiv ebx')
code.append('push eax')
code.append('pop eax')
code.append('ret')
return code
# 测试
expression = '1 + 2 * 3 - 4 / 2'
code = generate_code(expression)
print('\n'.join(code))
```
上面的代码实现了一个简单的四则运算表达式的编译器,将输入的中缀表达式转换成了汇编语言的中间代码。你可以根据具体需求对代码进行修改和扩展。
相关推荐
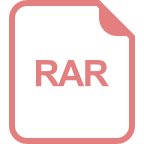
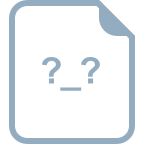














