C语言中缀式转后缀式代码
时间: 2023-08-18 20:25:15 浏览: 101
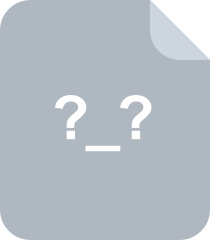
中缀转后缀_C语言代码
以下是C语言中缀式转后缀式的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 100
int get_priority(char op) {
switch (op) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
case '(':
case ')':
return 0;
default:
return -1;
}
}
void infix_to_postfix(char *infix, char *postfix) {
int i, j, top = 0;
char stack[MAX_SIZE], ch;
for (i = 0, j = 0; infix[i] != '\0'; i++) {
ch = infix[i];
if (ch == '(') {
stack[top++] = ch;
} else if (ch == ')') {
while (stack[top - 1] != '(') {
postfix[j++] = stack[--top];
}
top--;
} else if (ch >= '0' && ch <= '9') {
postfix[j++] = ch;
} else {
while (top > 0 && get_priority(stack[top - 1]) >= get_priority(ch)) {
postfix[j++] = stack[--top];
}
stack[top++] = ch;
}
}
while (top > 0) {
postfix[j++] = stack[--top];
}
postfix[j] = '\0';
}
int main() {
char infix[MAX_SIZE], postfix[MAX_SIZE];
printf("Enter an infix expression: ");
gets(infix);
infix_to_postfix(infix, postfix);
printf("Postfix expression: %s\n", postfix);
return 0;
}
```
该代码实现了一个简单的中缀式转后缀式的算法,可以将输入的中缀表达式转换为后缀表达式并输出。
阅读全文
相关推荐
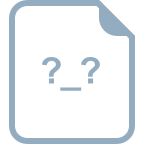



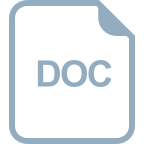
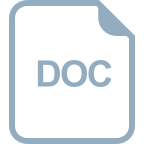
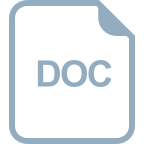
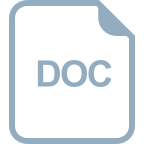






