c实现中缀表达式转后缀表达式代码
时间: 2024-06-13 08:04:14 浏览: 7
以下是C语言实现中缀表达式转后缀表达式的代码,同时也包含了计算后缀表达式的功能:
```
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define MAX_STACK_SIZE 100
typedef struct {
int top;
char items[MAX_STACK_SIZE];
} Stack;
void push(Stack *s, char c) {
if (s->top == MAX_STACK_SIZE - 1) {
printf("Stack is full\n");
exit(1);
}
s->items[++(s->top)] = c;
}
char pop(Stack *s) {
if (s->top == -1) {
printf("Stack is empty\n");
exit(1);
}
return s->items[(s->top)--];
}
int is_operator(char c) {
return (c == '+' || c == '-' || c == '*' || c == '/');
}
int precedence(char c) {
if (c == '+' || c == '-') {
return 1;
} else if (c == '*' || c == '/') {
return 2;
} else {
return 0;
}
}
void infix_to_postfix(char infix[], char postfix[]) {
Stack s;
s.top = -1;
int i = 0, j = 0;
char c, temp;
while ((c = infix[i++]) != '\0') {
if (isdigit(c)) {
postfix[j++] = c;
} else if (is_operator(c)) {
while (s.top != -1 && precedence(s.items[s.top]) >= precedence(c)) {
postfix[j++] = pop(&s);
}
push(&s, c);
} else if (c == '(') {
push(&s, c);
} else if (c == ')') {
while ((temp = pop(&s)) != '(') {
postfix[j++] = temp;
}
}
}
while (s.top != -1) {
postfix[j++] = pop(&s);
}
postfix[j] = '\0';
}
int evaluate_postfix(char postfix[]) {
Stack s;
s.top = -1;
int i = 0, op1, op2, result;
char c;
while ((c = postfix[i++]) != '\0') {
if (isdigit(c)) {
push(&s, c - '0');
} else if (is_operator(c)) {
op2 = pop(&s);
op1 = pop(&s);
switch (c) {
case '+':
result = op1 + op2;
break;
case '-':
result = op1 - op2;
break;
case '*':
result = op1 * op2;
break;
case '/':
result = op1 / op2;
break;
}
push(&s, result);
}
}
return pop(&s);
}
int main() {
char infix[100], postfix[100];
printf("Enter infix expression: ");
scanf("%s", infix);
infix_to_postfix(infix, postfix);
printf("Postfix expression: %s\n", postfix);
printf("Result: %d\n", evaluate_postfix(postfix));
return 0;
}
```
相关推荐
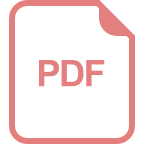
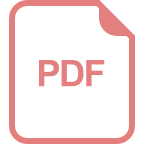














