C语言实现中缀表达式转后缀表达式
时间: 2023-12-22 12:04:26 浏览: 84
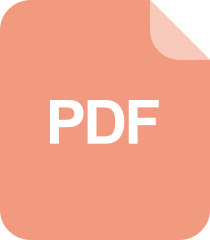
C语言实现中缀表达式转换为后缀表达式
C语言实现中缀表达式转后缀表达式的方法如下:
1. 创建一个空的栈,用于存储运算符。
2. 从左到右遍历中缀表达式的每个字符。
3. 如果遇到操作数(数字),直接输出到后缀表达式。
4. 如果遇到左括号"(",将其压入栈中。
5. 如果遇到右括号")",则将栈中的运算符依次弹出并输出到后缀表达式,直到遇到左括号为止。注意:左括号不输出到后缀表达式,也不入栈。
6. 如果遇到运算符,比较其与栈顶运算符的优先级:
- 如果栈为空,或栈顶运算符为左括号"(",则将当前运算符入栈。
- 如果当前运算符的优先级大于栈顶运算符的优先级,则将当前运算符入栈。
- 如果当前运算符的优先级小于或等于栈顶运算符的优先级,则将栈顶运算符弹出并输出到后缀表达式,然后继续比较当前运算符与新的栈顶运算符的优先级,直到当前运算符的优先级大于栈顶运算符的优先级,或栈为空,然后将当前运算符入栈。
7. 当遍历完中缀表达式后,将栈中剩余的运算符依次弹出并输出到后缀表达式。
以下是一个示例代码,演示了如何实现中缀表达式转后缀表达式的过程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 100
typedef struct {
char data[MAX_SIZE];
int top;
} Stack;
void initStack(Stack *s) {
s->top = -1;
}
int isEmpty(Stack *s) {
return s->top == -1;
}
int isFull(Stack *s) {
return s->top == MAX_SIZE - 1;
}
void push(Stack *s, char c) {
if (isFull(s)) {
printf("Stack is full.\n");
exit(1);
}
s->data[++s->top] = c;
}
char pop(Stack *s) {
if (isEmpty(s)) {
printf("Stack is empty.\n");
exit(1);
}
return s->data[s->top--];
}
int isOperator(char c) {
return c == '+' || c == '-' || c == '*' || c == '/';
}
int getPriority(char c) {
if (c == '*' || c == '/') {
return 2;
} else if (c == '+' || c == '-') {
return 1;
} else {
return 0;
}
}
void infixToPostfix(char *infix, char *postfix) {
Stack s;
initStack(&s);
int len = strlen(infix);
int j = 0;
for (int i = 0; i < len; i++) {
char c = infix[i];
if (isOperator(c)) {
while (!isEmpty(&s) && isOperator(s.data[s.top]) && getPriority(s.data[s.top]) >= getPriority(c)) {
postfix[j++] = pop(&s);
}
push(&s, c);
} else if (c == '(') {
push(&s, c);
} else if (c == ')') {
while (!isEmpty(&s) && s.data[s.top] != '(') {
postfix[j++] = pop(&s);
}
if (!isEmpty(&s) && s.data[s.top] == '(') {
pop(&s);
} else {
printf("Invalid expression: Unmatched parentheses.\n");
exit(1);
}
} else {
postfix[j++] = c;
}
}
while (!isEmpty(&s)) {
if (s.data[s.top] == '(') {
printf("Invalid expression: Unmatched parentheses.\n");
exit(1);
}
postfix[j++] = pop(&s);
}
postfix[j] = '\0';
}
int main() {
char infix[MAX_SIZE];
char postfix[MAX_SIZE];
printf("Enter an infix expression: ");
fgets(infix, MAX_SIZE, stdin);
infix[strlen(infix) - 1] = '\0';
infixToPostfix(infix, postfix);
printf("Postfix expression: %s\n", postfix);
return 0;
}
```
阅读全文
相关推荐




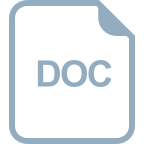
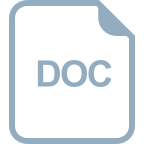







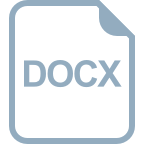