用c语言实现中缀表达式转后缀表达式、
时间: 2023-10-29 18:44:01 浏览: 62
下面是一个使用C语言实现中缀表达式转后缀表达式的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 100
// 定义栈结构
typedef struct {
char data[MAX_SIZE];
int top;
} Stack;
// 初始化栈
void initStack(Stack *s) {
s->top = -1;
}
// 判断栈是否为空
int isStackEmpty(Stack *s) {
return s->top == -1;
}
// 判断栈是否已满
int isStackFull(Stack *s) {
return s->top == MAX_SIZE - 1;
}
// 入栈
void push(Stack *s, char value) {
if (isStackFull(s)) {
printf("Stack is full.\n");
return;
}
s->data[++(s->top)] = value;
}
// 出栈
char pop(Stack *s) {
if (isStackEmpty(s)) {
printf("Stack is empty.\n");
return '\0';
}
return s->data[(s->top)--];
}
// 获取栈顶元素
char peek(Stack *s) {
if (isStackEmpty(s)) {
printf("Stack is empty.\n");
return '\0';
}
return s->data[s->top];
}
// 判断运算符的优先级
int getPriority(char operator) {
switch (operator) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
case '^':
return 3;
default:
return 0;
}
}
// 中缀表达式转后缀表达式
void infixToPostfix(char *infix, char *postfix) {
Stack operatorStack;
initStack(&operatorStack);
int i, j;
char ch, topOperator;
for (i = 0, j = 0; infix[i] != '\0'; i++) {
ch = infix[i];
if (ch >= 'a' && ch <= 'z') { // 如果是操作数,直接输出到后缀表达式中
postfix[j++] = ch;
} else if (ch == '(') { // 如果是左括号,入栈
push(&operatorStack, ch);
} else if (ch == ')') { // 如果是右括号,将栈中的运算符弹出并输出,直到遇到左括号
while ((topOperator = pop(&operatorStack)) != '(') {
postfix[j++] = topOperator;
}
} else if (ch == '+' || ch == '-' || ch == '*' || ch == '/' || ch == '^') { // 如果是运算符
// 将优先级比当前运算符高或者相等的运算符弹出并输出
while (!isStackEmpty(&operatorStack) && peek(&operatorStack) != '(' &&
getPriority(ch) <= getPriority(peek(&operatorStack))) {
postfix[j++] = pop(&operatorStack);
}
push(&operatorStack, ch); // 将当前运算符入栈
}
}
// 将栈中剩余的运算符弹出并输出
while (!isStackEmpty(&operatorStack)) {
postfix[j++] = pop(&operatorStack);
}
postfix[j] = '\0'; // 结尾加上字符串结束符
}
int main() {
char infix[MAX_SIZE];
char postfix[MAX_SIZE];
printf("Enter an infix expression: ");
fgets(infix, sizeof(infix), stdin);
infix[strlen(infix) - 1] = '\0'; // 去掉末尾的换行符
infixToPostfix(infix, postfix);
printf("Postfix expression: %s\n", postfix);
return 0;
}
```
这个示例代码实现了一个简单的中缀表达式转后缀表达式的程序。运行代码后,用户需要输入一个中缀表达式,程序会将其转换为后缀表达式并输出。
注意,在这个示例中,假设输入的中缀表达式合法且只包含小写字母、运算符和括号。如果输入的中缀表达式有语法错误或不符合规范,程序可能会产生错误的输出。在实际的应用中,可能需要对输入进行更严格的验证和错误处理。
希望这个示例能帮助你理解如何使用C语言实现中缀表达式转后缀表达式!
相关推荐
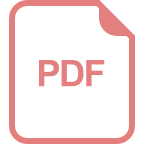
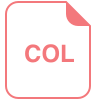
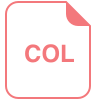
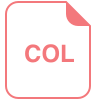
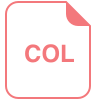










