C语言如何实现中缀表达式转后缀表达式?
时间: 2024-06-14 13:04:00 浏览: 16
以下是C语言实现中缀表达式转后缀表达式的具体代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX 50
typedef struct {
char data[MAX];
int top;
} SeqStack;
void InitStack(SeqStack *s) {
s->top = -1;
}
int StackEmpty(SeqStack *s) {
return s->top == -1 ? 1 : 0;
}
int Push(SeqStack *s, char x) {
if (s->top == MAX - 1) {
return 0;
}
s->top++;
s->data[s->top] = x;
return 1;
}
int Pop(SeqStack *s, char *x) {
if (s->top == -1) {
return 0;
}
*x = s->data[s->top];
s->top--;
return 1;
}
int GetTop(SeqStack *s, char *x) {
if (s->top == -1) {
return 0;
}
*x = s->data[s->top];
return 1;
}
int Priority(char x) {
switch (x) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
default:
return 0;
}
}
void InfixToPostfix(char *infix, char *postfix) {
SeqStack s;
InitStack(&s);
char x, y;
int i = 0, j = 0;
while (infix[i] != '\0') {
switch (infix[i]) {
case '(':
Push(&s, infix[i]);
i++;
break;
case ')':
Pop(&s, &x);
while (x != '(') {
postfix[j++] = x;
Pop(&s, &x);
}
i++;
break;
case '+':
case '-':
case '*':
case '/':
while (!StackEmpty(&s) && Priority(infix[i]) <= Priority(s.data[s.top])) {
Pop(&s, &x);
postfix[j++] = x;
}
Push(&s, infix[i]);
i++;
break;
default:
while (infix[i] >= '0' && infix[i] <= '9') {
postfix[j++] = infix[i];
i++;
}
postfix[j++] = '#'; // 用#分隔两个数字
}
}
while (!StackEmpty(&s)) {
Pop(&s, &x);
postfix[j++] = x;
}
postfix[j] = '\0';
}
int main() {
char infix[MAX], postfix[MAX];
printf("请输入中缀表达式:");
scanf("%s", infix);
InfixToPostfix(infix, postfix);
printf("后缀表达式为:%s\n", postfix);
return 0;
}
```
相关推荐
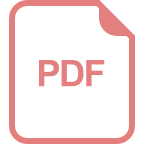














