中缀表达式转换为后缀表达式输出,并求后级表达式的值,用c++解决
时间: 2024-12-15 22:12:55 浏览: 23
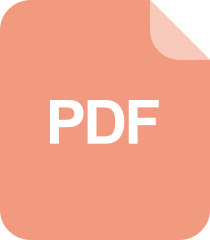
string 中缀表达式求值

中缀表达式(也称为前缀表达式)通常包含运算符和操作数,例如 "A + B * C"。将其转换为等效的后缀表达式(也称作逆波兰表达式),就是把运算符放在其操作数之后。这个过程可以采用堆栈数据结构来实现,比如Shunting Yard算法。
在C++中,你可以按照以下步骤来处理这个问题:
1. 定义一个字符数组来存储输入的中缀表达式,一个整型数组用于存放后缀表达式,以及两个指针分别表示中缀表达式的当前位置和后缀表达式的开始位置。
2. 遍历中缀表达式,遇到数字就直接添加到后缀表达式数组;遇到左括号则压入堆栈;遇到右括号则将堆栈中的运算符依次弹出并添加到后缀表达式数组,直到遇到左括号为止。
3. 遇到运算符时,如果堆栈为空或堆栈顶的运算符优先级小于当前运算符,将当前运算符压入堆栈;否则,将堆栈顶的运算符和其对应的操作数弹出,然后将当前运算符压入堆栈。
4. 最后遍历结束后,堆栈中的剩余运算符和操作数依次添加到后缀表达式数组。
5. 对于求解后缀表达式的值,你需要再创建一个堆栈来计算结果。从后缀表达式数组的末尾开始,读取每一个元素,如果是数字就直接相加到结果上,如果是运算符则从堆栈取出相应的操作数进行运算,最后堆栈只有一个元素即为结果。
下面是简化版的C++代码框架示例:
```cpp
#include <iostream>
#include <stack>
#include <string>
// 帮助函数,判断运算符优先级
int compareOperands(char op1, char op2) {
// ... (根据实际需要的优先级规则实现)
}
std::string infixToPostfix(const std::string& infixExp) {
std::stack<char> opStack;
std::string postfixExp = "";
for (char c : infixExp) {
if (isdigit(c)) {
postfixExp += c;
} else if (c == '(') {
opStack.push(c);
} else if (c == ')') {
while (!opStack.empty() && opStack.top() != '(') {
postfixExp += opStack.pop();
}
if (!opStack.empty()) {
opStack.pop(); // 弹出左括号
}
} else {
while (!opStack.empty() && compareOperands(opStack.top(), c) >= 0) {
postfixExp += opStack.pop();
}
opStack.push(c); // 将运算符压入堆栈
}
}
while (!opStack.empty()) {
postfixExp += opStack.pop();
}
return postfixExp;
}
int evaluatePostfix(const std::string& postfixExp) {
std::stack<int> numStack;
for (char c : postfixExp) {
if (isdigit(c)) {
numStack.push(c - '0'); // 转换为整数
} else {
int b = numStack.top(); // 取第二个操作数
numStack.pop();
int a = numStack.top(); // 取第一个操作数
numStack.pop();
switch (c) {
case '+':
numStack.push(a + b);
break;
// 添加其他运算符的case...
}
}
}
return numStack.top();
}
int main() {
std::string infixExp = "A+B*C";
std::string postfixExp = infixToPostfix(infixExp);
int result = evaluatePostfix(postfixExp);
std::cout << "后缀表达式: " << postfixExp << "\n";
std::cout << "计算结果: " << result << "\n";
return 0;
}
```
注意,这只是一个简化的版本,实际项目中可能需要处理更复杂的情况,如括号嵌套、支持更多运算符等。同时,`compareOperands()` 函数需要根据具体的运算符优先级规则来实现。
阅读全文
相关推荐
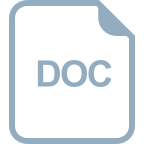
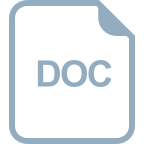

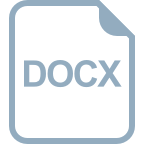










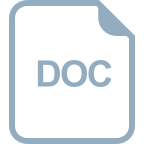
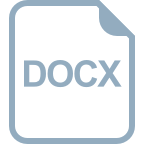
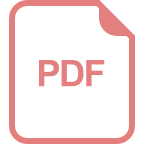
