请用Python写一个json格式转换成 mat格式的代码
时间: 2023-09-06 12:05:12 浏览: 127
### 回答1:
您可以使用Python的`json`库和`scipy`库来实现这个功能。代码示例如下:
```
import json
import scipy.io as sio
# 读取json文件
with open('data.json', 'r') as file:
data = json.load(file)
# 保存为mat文件
sio.savemat('data.mat', {'data': data})
```
上面的代码会读取名为`data.json`的json文件,并将其保存为名为`data.mat`的mat文件。
### 回答2:
以下是一个将JSON格式转换成MAT格式的Python代码示例:
```python
import json
import scipy.io as sio
def json_to_mat(json_file, mat_file):
with open(json_file, 'r') as f:
data = json.load(f)
sio.savemat(mat_file, data)
# 示例用法
json_file = 'data.json'
mat_file = 'data.mat'
json_to_mat(json_file, mat_file)
```
在这个示例中,我们首先通过`json.load()`函数将JSON文件加载为一个Python字典或列表。然后,我们使用`scipy.io.savemat()`函数将数据保存到MAT文件中。
请确保已经安装了`scipy`库,在终端中运行以下命令进行安装:
```shell
pip install scipy
```
在使用上述代码时,需要将`json_file`替换为实际的JSON文件路径,将`mat_file`替换为您希望保存MAT文件的路径。这样,您就可以将JSON文件转换为MAT文件格式了。
### 回答3:
以下是一个用Python编写的将JSON格式转换为mat格式的示例代码:
```python
import json
import scipy.io
def json_to_mat(json_file, mat_file):
with open(json_file, 'r') as f:
data = json.load(f)
scipy.io.savemat(mat_file, data)
print("转换完成!")
json_file = 'input.json'
mat_file = 'output.mat'
json_to_mat(json_file, mat_file)
```
代码中的`json_file`为输入的JSON文件路径,`mat_file`为输出的MAT文件路径。首先读取JSON文件中的数据,然后使用`scipy.io.savemat()`函数将数据保存为MAT文件。
请将代码中的`input.json`替换为你要转换的JSON文件路径,将`output.mat`替换为你想保存的MAT文件路径,运行代码即可将JSON格式数据转换为MAT格式并保存为指定文件。
相关推荐
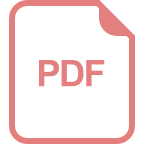
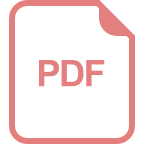









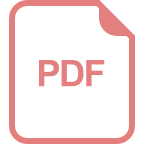
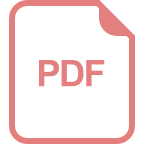
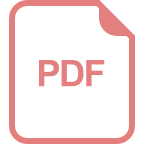
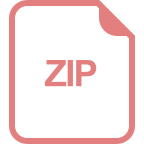