mobilenetv3 exp size
时间: 2023-10-30 08:09:39 浏览: 105
MobileNetV3 的扩张因子(expansion factor)是指在每个深度可分离卷积层中,输入通道数与输出通道数之间的比例。MobileNetV3 中的扩张因子可以取 1 或大于 1 的整数,用于控制模型的宽度。例如,当扩张因子为 6 时,输出通道数是输入通道数的 6 倍。
MobileNetV3 的扩张因子可以应用在两个地方:第一个是在瓶颈块(bottleneck block)中,第二个是在最后的卷积层中。在瓶颈块中,扩张因子被应用于第二个 1x1 卷积层的输入和输出通道数之间的比例。在最后的卷积层中,扩张因子被应用于输入通道数和输出通道数之间的比例。
MobileNetV3 的扩张因子可以通过调整超参数来进行控制。在 TensorFlow 中,可以通过设置 `expansion_factor` 参数来指定扩张因子。默认情况下,`expansion_factor` 的值为 6。
相关问题
mobilenetv3与yolov5结合代码
将MobileNetV3和YOLOv5结合起来需要进行以下步骤:
1. 首先,需要下载YOLOv5的代码和预训练权重,以及MobileNetV3的代码和预训练权重。
2. 接着,需要在YOLOv5中修改模型结构,以便将MobileNetV3作为YOLOv5的特征提取器。可以在YOLOv5的models/yolov5s.py文件中进行修改,将原来的卷积层替换为MobileNetV3的卷积层。
3. 在训练时,需要将YOLOv5的训练数据集进行转换,以适应MobileNetV3的输入尺寸。可以使用YOLOv5的datasets.py文件中的resize方法进行转换。
4. 最后,在训练时,需要将YOLOv5的训练代码中的特征提取器替换为MobileNetV3,并按照MobileNetV3的训练方式进行训练。
下面是一个简单的参考代码,仅供参考:
```python
import torch
import torch.nn as nn
import torchvision.models as models
from models.common import Conv, DWConv
class MobileNetV3(nn.Module):
def __init__(self, width_mult=1.0):
super(MobileNetV3, self).__init__()
self.inplanes = 16
self.cfgs = [
# k, exp, c, se, nl, s,
[3, 16, 16, False, 'relu', 1],
[3, 64, 24, False, 'relu', 2],
[3, 72, 24, False, 'relu', 1],
[5, 72, 40, True, 'relu', 2],
[5, 120, 40, True, 'relu', 1],
[5, 120, 40, True, 'relu', 1],
[3, 240, 80, False, 'hswish', 2],
[3, 200, 80, False, 'hswish', 1],
[3, 184, 80, False, 'hswish', 1],
[3, 184, 80, False, 'hswish', 1],
[3, 480, 112, True, 'hswish', 1],
[3, 672, 112, True, 'hswish', 1],
[5, 672, 160, True, 'hswish', 2],
[5, 960, 160, True, 'hswish', 1],
[5, 960, 160, True, 'hswish', 1],
]
# head
self.conv1 = Conv(3, self.inplanes, kernel_size=3, stride=2, padding=1, bias=False)
self.bn1 = nn.BatchNorm2d(self.inplanes)
self.hswish = Hswish(inplace=True)
# body
self.features = nn.ModuleList([])
for k, exp, c, se, nl, s in self.cfgs:
outplanes = int(c * width_mult)
self.features.append(InvertedResidual(self.inplanes, outplanes, k, s, exp, se, nl))
self.inplanes = outplanes
# tail
self.conv2 = Conv(self.inplanes, 960, kernel_size=1, stride=1, padding=0, bias=False)
self.bn2 = nn.BatchNorm2d(960)
self.hswish2 = Hswish(inplace=True)
def forward(self, x):
# head
x = self.conv1(x)
x = self.bn1(x)
x = self.hswish(x)
# body
for f in self.features:
x = f(x)
# tail
x = self.conv2(x)
x = self.bn2(x)
x = self.hswish2(x)
return x
class YOLOv5(nn.Module):
def __init__(self, num_classes=80):
super(YOLOv5, self).__init__()
self.head = Conv(960, 1024, 3, stride=1, padding=1)
self.body = nn.Sequential(
Residual(1024, 512, 1),
Conv(512, 256, 1),
nn.Upsample(scale_factor=2, mode='nearest'),
Residual(512, 256, 1),
Conv(256, 128, 1),
nn.Upsample(scale_factor=2, mode='nearest'),
Residual(256, 128, 1),
Conv(128, 64, 1),
nn.Upsample(scale_factor=2, mode='nearest'),
Residual(128, 64, 1),
)
self.tail = nn.Conv2d(64, 3 * (5 + num_classes), 1)
def forward(self, x):
x = self.head(x)
x = self.body(x)
x = self.tail(x)
return x
if __name__ == '__main__':
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
# load MobileNetV3
mobilenetv3 = MobileNetV3().to(device)
mobilenetv3.load_state_dict(torch.load('mobilenetv3_weights.pth'))
# load YOLOv5
yolov5 = YOLOv5().to(device)
yolov5.load_state_dict(torch.load('yolov5_weights.pth'))
# replace feature extractor of YOLOv5 with MobileNetV3
yolov5.head = mobilenetv3.conv2
yolov5.body = mobilenetv3.features
# train YOLOv5 with MobileNetV3 feature extractor
train_dataset = ...
train_loader = ...
optimizer = ...
loss_fn = ...
for epoch in range(num_epochs):
for batch_idx, (data, targets) in enumerate(train_loader):
data, targets = data.to(device), targets.to(device)
optimizer.zero_grad()
outputs = yolov5(data)
loss = loss_fn(outputs, targets)
loss.backward()
optimizer.step()
```
yolov5怎么使用MobileNetv3的预训练模型
在使用 YOLOv5 时,可以使用 MobileNetv3 的预训练模型来进行目标检测任务。下面是使用 MobileNetv3 的预训练模型的步骤:
1. 下载 MobileNetv3 的预训练模型文件,可以从以下链接中下载:
- https://github.com/d-li14/mobilenetv3.pytorch/releases/download/v1.0/mobilenetv3_large_1.0-7d1d638a.pth
- https://github.com/d-li14/mobilenetv3.pytorch/releases/download/v1.0/mobilenetv3_small_1.0-23cdd98c.pth
2. 将下载的预训练模型文件放置在 YOLOv5 的 `weights` 文件夹中。
3. 修改 YOLOv5 的配置文件 `yolov5s.yaml`,将 `backbone` 中的 `name` 修改为 `mobilenetv3_large`.
4. 运行 YOLOv5 的 `train.py` 脚本,指定数据集、预训练模型文件以及其他参数,例如:
```python
python train.py --img 640 --batch 16 --epochs 100 --data coco.yaml --weights weights/mobilenetv3_large_1.0-7d1d638a.pth
```
其中,`--data` 参数指定数据集的配置文件,`--weights` 参数指定预训练模型文件的路径。
5. 训练完成后,可以使用 YOLOv5 的 `detect.py` 脚本进行目标检测。需要注意的是,在 `detect.py` 中也需要将 `backbone` 中的 `name` 修改为 `mobilenetv3_large`。
```python
python detect.py --source test.jpg --weights runs/train/exp/weights/best.pt --img-size 640 --conf 0.4 --backbone-name mobilenetv3_large
```
其中,`--source` 参数指定输入图像的路径,`--weights` 参数指定训练得到的模型文件的路径,`--img-size` 参数指定输入图像的大小,`--conf` 参数指定置信度阈值,`--backbone-name` 参数指定使用的 backbone 模型名称。
阅读全文
相关推荐
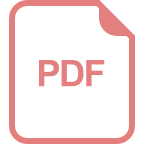
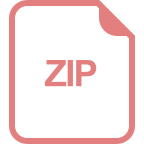


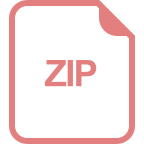
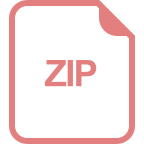
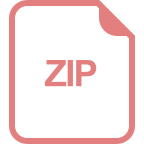
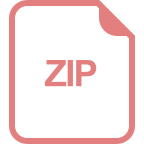
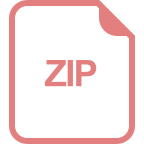
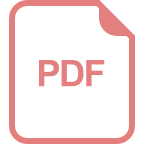