springboot 集成mmap读写文件
时间: 2024-01-11 08:22:12 浏览: 119
在Spring Boot中集成mmap读写文件可以通过以下步骤实现:
1. 引入依赖:在`pom.xml`文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
```
2. 创建文件操作工具类:创建一个`FileUtils`工具类,用于进行文件的读写操作。在该类中,可以使用Java的`MappedByteBuffer`类来实现mmap读写文件的功能。以下是一个简单的示例代码:
```java
import java.io.File;
import java.io.RandomAccessFile;
import java.nio.MappedByteBuffer;
import java.nio.channels.FileChannel;
public class FileUtils {
public static void writeFileWithMmap(String filePath, String content) throws Exception {
File file = new File(filePath);
RandomAccessFile randomAccessFile = new RandomAccessFile(file, "rw");
FileChannel fileChannel = randomAccessFile.getChannel();
MappedByteBuffer mappedByteBuffer = fileChannel.map(FileChannel.MapMode.READ_WRITE, 0, content.length());
mappedByteBuffer.put(content.getBytes());
fileChannel.close();
randomAccessFile.close();
}
public static String readFileWithMmap(String filePath) throws Exception {
File file = new File(filePath);
RandomAccessFile randomAccessFile = new RandomAccessFile(file, "r");
FileChannel fileChannel = randomAccessFile.getChannel();
MappedByteBuffer mappedByteBuffer = fileChannel.map(FileChannel.MapMode.READ_ONLY, 0, file.length());
byte[] bytes = new byte[(int) file.length()];
mappedByteBuffer.get(bytes);
fileChannel.close();
randomAccessFile.close();
return new String(bytes);
}
}
```
3. 在Spring Boot应用中使用mmap读写文件:在需要使用mmap读写文件的地方,可以直接调用`FileUtils`工具类中的方法进行文件的读写操作。以下是一个简单的示例代码:
```java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application {
public static void main(String[] args) throws Exception {
SpringApplication.run(Application.class, args);
String filePath = "path/to/file.txt";
String content = "Hello, mmap!";
// 写文件
FileUtils.writeFileWithMmap(filePath, content);
// 读文件
String fileContent = FileUtils.readFileWithMmap(filePath);
System.out.println(fileContent);
}
}
```
请注意,以上示例代码仅为演示目的,实际使用时需要根据具体需求进行适当的修改和优化。
阅读全文
相关推荐
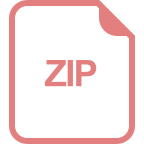
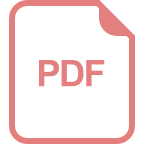
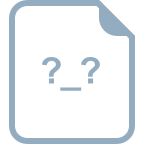
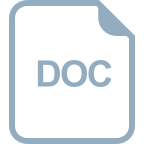
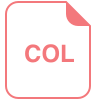










