linux mmap映射文件,映射的key=value的文件,后从映射文件读取数据, 代码实现
时间: 2024-03-08 19:46:02 浏览: 146
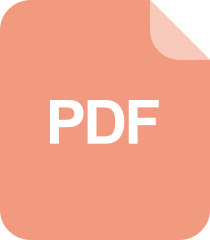
linux下的内存映射函数mmap详解及示例代码.pdf

以下是一个简单的例子,演示了如何使用`mmap()`在Linux中映射一个文件,并读取其中的内容:
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/mman.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <string.h>
#define FILESIZE 1024
int main(int argc, char *argv[]) {
int fd;
char *addr;
struct stat sb;
// 打开文件
fd = open("key_value.txt", O_RDONLY);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
// 获取文件的大小
if (fstat(fd, &sb) == -1) {
perror("fstat");
exit(EXIT_FAILURE);
}
// 映射文件
addr = mmap(NULL, sb.st_size, PROT_READ, MAP_PRIVATE, fd, 0);
if (addr == MAP_FAILED) {
perror("mmap");
exit(EXIT_FAILURE);
}
// 读取文件内容
char *key = strtok(addr, "=");
char *value = strtok(NULL, "\n");
while (key && value) {
printf("key: %s, value: %s\n", key, value);
key = strtok(NULL, "=");
value = strtok(NULL, "\n");
}
// 解除映射
if (munmap(addr, sb.st_size) == -1) {
perror("munmap");
exit(EXIT_FAILURE);
}
// 关闭文件
if (close(fd) == -1) {
perror("close");
exit(EXIT_FAILURE);
}
return 0;
}
```
假设我们有一个名为`key_value.txt`的文件,其中包含以下内容:
```
name=John
age=30
location=USA
```
这个程序将读取该文件,并将每行解析为键值对。在这种情况下,它将输出:
```
key: name, value: John
key: age, value: 30
key: location, value: USA
```
阅读全文
相关推荐




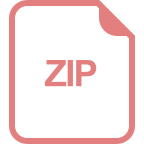
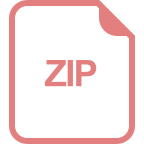
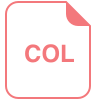
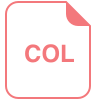
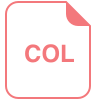
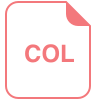
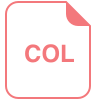
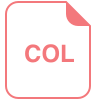
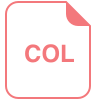
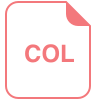
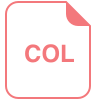
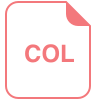