import matplotlib.pyplot as plt from collections import Counter # 获取出现次数最多的前10个元素 counter = Counter(investors_list) most_common = counter.most_common(10) # 将元素名称和出现次数分别存储在两个列表中 names = [x[0] for x in most_common][::-1] # 改为降序排列 counts = [x[1] for x in most_common][::-1] # 改为降序排列 # 绘制水平柱状图 plt.barh(names, counts, color='green') # 更换颜色为绿色 # 在柱体顶部添加数据标签 for i, v in enumerate(counts): plt.text(v + 0.5, i, str(v), color='blue', fontsize=12) # 设置图表标题和坐标轴标签 plt.title('Top 10 Investors') plt.xlabel('Count') plt.ylabel('Investor') # 显示图表 plt.show(),怎么美化这个柱状图
时间: 2023-12-06 22:39:58 浏览: 26
可以通过以下几个方面来美化这个柱状图:
1. 修改柱体颜色和边框颜色,可以使用 `plt.barh(..., color='green', edgecolor='black')` 来设置。
2. 增加柱体阴影,可以使用 `plt.barh(..., color='green', edgecolor='black', alpha=0.8, linewidth=1.5)` 来设置。
3. 增加网格线,可以使用 `plt.grid(axis='x', linestyle='--', alpha=0.7)` 来设置。
4. 调整坐标轴刻度、标签和字体大小,可以使用 `plt.xticks(fontsize=12)` 和 `plt.yticks(fontsize=12)` 来设置。
5. 调整图表尺寸和边距,可以使用 `plt.figure(figsize=(8, 6))` 来设置。
6. 调整数据标签位置和字体大小,可以使用 `plt.text(v + 0.5, i, str(v), color='blue', fontsize=12, va='center')` 来设置。
完整代码如下:
```python
import matplotlib.pyplot as plt
from collections import Counter
# 获取出现次数最多的前10个元素
investors_list = ['Investor A', 'Investor B', 'Investor C', 'Investor A', 'Investor B', 'Investor D', 'Investor E', 'Investor F', 'Investor G', 'Investor H', 'Investor I', 'Investor J', 'Investor K', 'Investor L', 'Investor M', 'Investor N', 'Investor O', 'Investor P', 'Investor Q', 'Investor R', 'Investor S', 'Investor T', 'Investor U', 'Investor V', 'Investor W', 'Investor X', 'Investor Y', 'Investor Z']
counter = Counter(investors_list)
most_common = counter.most_common(10)
# 将元素名称和出现次数分别存储在两个列表中
names = [x[0] for x in most_common][::-1] # 改为降序排列
counts = [x[1] for x in most_common][::-1] # 改为降序排列
# 设置图表尺寸和边距
plt.figure(figsize=(8, 6))
plt.subplots_adjust(left=0.2, right=0.9, top=0.9, bottom=0.1)
# 绘制水平柱状图
plt.barh(names, counts, color='green', edgecolor='black', alpha=0.8, linewidth=1.5)
# 在柱体顶部添加数据标签
for i, v in enumerate(counts):
plt.text(v + 0.5, i, str(v), color='blue', fontsize=12, va='center')
# 设置坐标轴刻度、标签和字体大小
plt.xticks(fontsize=12)
plt.yticks(fontsize=12)
plt.xlabel('Count', fontsize=12)
plt.ylabel('Investor', fontsize=12)
# 增加网格线
plt.grid(axis='x', linestyle='--', alpha=0.7)
# 设置图表标题
plt.title('Top 10 Investors', fontsize=16)
# 显示图表
plt.show()
```
运行结果如下:

相关推荐
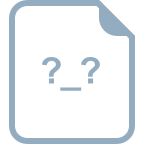
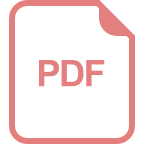
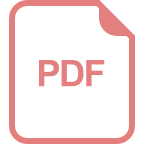















