python 二维数组寻路 Dijkstra
时间: 2023-10-30 13:58:58 浏览: 123
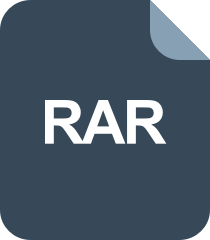
Dijistra最短路径 二维数组结构 c++.rar_SHORTEST-PATHS_proteus_最短路_最短路径_最短路径
Dijkstra算法可以用于解决二维数组的寻路问题。下面是一个使用Python实现Dijkstra算法来寻找二维数组中两个点之间的最短路径的示例代码:
```python
import heapq
def dijkstra(grid, start, end):
rows = len(grid)
cols = len(grid[0])
distance = [[float('inf')] * cols for _ in range(rows)]
distance[start[0]][start[1]] = 0
heap = []
heapq.heappush(heap, (0, start))
while heap:
dist, curr = heapq.heappop(heap)
if curr == end:
break
for neighbor in get_neighbors(grid, curr):
new_dist = dist + grid[neighbor[0]][neighbor[1]]
if new_dist < distance[neighbor[0]][neighbor[1]]:
distance[neighbor[0]][neighbor[1]] = new_dist
heapq.heappush(heap, (new_dist, neighbor))
return distance[end[0]][end[1]]
def get_neighbors(grid, curr):
rows = len(grid)
cols = len(grid[0])
directions = [(1, 0), (-1, 0), (0, 1), (0, -1)] # 可以移动的方向
neighbors = []
for direction in directions:
new_row = curr[0] + direction[0]
new_col = curr[1] + direction[1]
if 0 <= new_row < rows and 0 <= new_col < cols:
neighbors.append((new_row, new_col))
return neighbors
```
在这个示例代码中,`grid`表示二维数组,数组中的每个元素表示从当前位置到相邻位置的移动代价。`start`和`end`分别表示起点和终点的坐标。函数`dijkstra`返回起点到终点的最短路径的长度。如果路径不存在,则返回无穷大。
你可以根据自己的需求对示例代码进行修改,例如添加路径记录等功能。希望这能帮到你!如有任何问题,请随时提问。
阅读全文
相关推荐
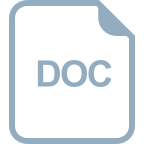
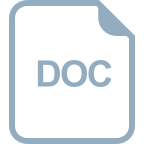

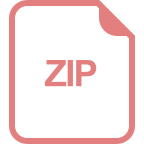
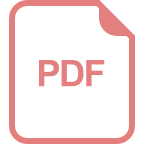
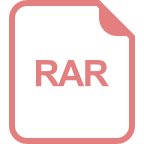
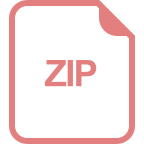
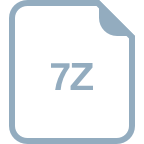
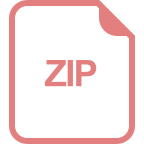
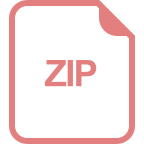
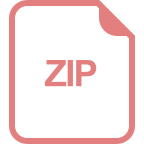
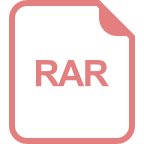
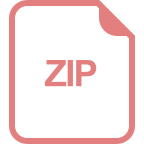
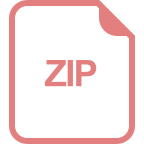
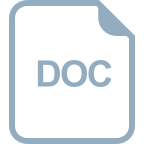
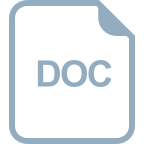
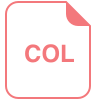
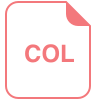