用Python写一个俄罗斯方块游戏代码
时间: 2024-01-01 17:03:14 浏览: 102
当然可以!以下是一个俄罗斯方块游戏的Python代码:
```
import pygame
import random
# 设置窗口大小
screen_width = 320
screen_height = 480
# 初始化 Pygame
pygame.init()
# 创建窗口
screen = pygame.display.set_mode((screen_width, screen_height))
# 游戏标题
pygame.display.set_caption('俄罗斯方块')
# 颜色定义(RGB格式)
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
YELLOW = (255, 255, 0)
# 方块大小定义
block_size = 20
# 定义方块的形状
tetrominoes = [
[[1, 1, 1],
[0, 1, 0]],
[[0, 2, 2],
[2, 2, 0]],
[[3, 3, 0],
[0, 3, 3]],
[[4, 0, 0],
[4, 4, 4]],
[[0, 0, 5],
[5, 5, 5]],
[[6, 6, 6, 6]],
[[7, 7],
[7, 7]],
]
# 定义方块的颜色
block_colors = [
WHITE,
YELLOW,
BLUE,
RED,
GREEN,
# PURPLE
(255,0,255),
# ORANGE
(255,165,0)
]
# 获取一个新的方块
def new_block():
block = random.choice(tetrominoes)
color = random.choice(block_colors)
return block, color
# 绘制方块
def draw_block(x, y, color):
pygame.draw.rect(screen, color, (x * block_size, y * block_size, block_size, block_size))
pygame.draw.rect(screen, BLACK, (x * block_size, y * block_size, block_size, block_size), 2)
# 检查方块是否合法
def check_piece_valid(piece, x, y):
try:
for row in range(len(piece)):
for col in range(len(piece[0])):
if piece[row][col] == 0:
continue
if x + col < 0 or x + col >= screen_width // block_size:
return False
if y + row < 0 or y + row >= screen_height // block_size:
return False
return True
except IndexError:
return False
# 游戏循环
def game_loop():
# 初始位置
current_x, current_y = screen_width // block_size // 2, 0
# 获取新的方块和颜色
current_piece, current_color = new_block()
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
# 检查是否可以下落
if check_piece_valid(current_piece, current_x, current_y + 1):
current_y += 1
else:
# 不再可以下落,将方块固定在当前位置
for row in range(len(current_piece)):
for col in range(len(current_piece[0])):
if current_piece[row][col] != 0:
draw_block(current_x + col, current_y + row, current_color)
# 获取新的方块和颜色
current_piece, current_color = new_block()
current_x, current_y = screen_width // block_size // 2, 0
# 绘制当前方块
screen.fill(BLACK)
for row in range(len(current_piece)):
for col in range(len(current_piece[0])):
if current_piece[row][col] != 0:
draw_block(current_x + col, current_y + row, current_color)
# 设置帧率
pygame.display.set_caption('俄罗斯方块(帧率:' + str(int(clock.get_fps())) + ')')
# 更新屏幕
pygame.display.update()
# 设置帧率
clock.tick(25)
# 启动游戏
clock = pygame.time.Clock()
game_loop()
```
阅读全文
相关推荐
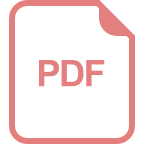









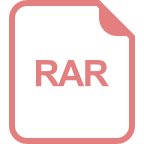
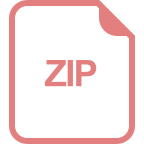