给我用python写一个俄罗斯方块的代码
时间: 2023-12-31 17:05:27 浏览: 79
好的,以下是一个简单的俄罗斯方块游戏的Python代码:
```
import pygame
import random
# 定义窗口大小
WINDOW_WIDTH = 400
WINDOW_HEIGHT = 500
# 定义方块尺寸
BLOCK_SIZE = 20
# 定义方块位置
BLOCK_X = WINDOW_WIDTH // 2 - BLOCK_SIZE // 2
BLOCK_Y = BLOCK_SIZE
# 定义方块类型和颜色
BLOCK_TYPE = ['I', 'J', 'L', 'O', 'S', 'T', 'Z']
BLOCK_COLOR = [(0, 255, 255), (0, 0, 255), (255, 165, 0), (255, 255, 0), (0, 255, 0), (128, 0, 128), (255, 0, 0)]
# 定义方块形状
BLOCK_SHAPE = {
'I': [[1, 1, 1, 1]],
'J': [[0, 1, 0], [0, 1, 0], [1, 1, 0]],
'L': [[0, 1, 0], [0, 1, 0], [0, 1, 1]],
'O': [[1, 1], [1, 1]],
'S': [[0, 1, 1], [1, 1, 0]],
'T': [[0, 1, 0], [1, 1, 1], [0, 0, 0]],
'Z': [[1, 1, 0], [0, 1, 1]]
}
# 初始化pygame
pygame.init()
# 创建窗口
window = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
# 设置游戏标题
pygame.display.set_caption('俄罗斯方块')
# 设置游戏时钟
clock = pygame.time.Clock()
# 定义游戏状态
game_over = False
# 定义方块变量
block_type = random.choice(BLOCK_TYPE)
block_color = BLOCK_COLOR[BLOCK_TYPE.index(block_type)]
block_shape = BLOCK_SHAPE[block_type]
block_x = BLOCK_X
block_y = BLOCK_Y
# 定义方块变量
shadow_type = block_type
shadow_color = (100, 100, 100)
shadow_shape = block_shape
shadow_x = block_x
shadow_y = block_y
# 定义方块函数
def draw_block(x, y, shape, color):
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j] == 1:
pygame.draw.rect(window, color, (x + j * BLOCK_SIZE, y + i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
pygame.draw.rect(window, (0, 0, 0), (x + j * BLOCK_SIZE, y + i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE), 1)
# 定义影子函数
def draw_shadow(x, y, shape, color):
shadow_y = y
while not hit_wall(x, shadow_y + BLOCK_SIZE, shape):
shadow_y += BLOCK_SIZE
draw_block(x, shadow_y - BLOCK_SIZE, shape, color)
# 定义检查函数
def hit_wall(x, y, shape):
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j] == 1:
if x + j * BLOCK_SIZE < 0 or x + j * BLOCK_SIZE >= WINDOW_WIDTH or y + i * BLOCK_SIZE >= WINDOW_HEIGHT:
return True
return False
def hit_block(x, y, shape, block_list):
for block in block_list:
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j] == 1 and block.x == x + j * BLOCK_SIZE and block.y == y + i * BLOCK_SIZE:
return True
return False
# 定义消行函数
def clear_block(block_list):
row_dict = {}
for block in block_list:
if block.y not in row_dict:
row_dict[block.y] = [block]
else:
row_dict[block.y].append(block)
for row in row_dict:
if len(row_dict[row]) == 10:
for block in row_dict[row]:
block_list.remove(block)
for block in block_list:
if block.y < row:
block.y += BLOCK_SIZE
# 定义游戏主循环
while not game_over:
# 清屏
window.fill((255, 255, 255))
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
# 处理键盘事件
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT] and not hit_wall(block_x - BLOCK_SIZE, block_y, block_shape) and not hit_block(block_x - BLOCK_SIZE, block_y, block_shape, block_list):
block_x -= BLOCK_SIZE
if keys[pygame.K_RIGHT] and not hit_wall(block_x + BLOCK_SIZE, block_y, block_shape) and not hit_block(block_x + BLOCK_SIZE, block_y, block_shape, block_list):
block_x += BLOCK_SIZE
if keys[pygame.K_DOWN] and not hit_wall(block_x, block_y + BLOCK_SIZE, block_shape) and not hit_block(block_x, block_y + BLOCK_SIZE, block_shape, block_list):
block_y += BLOCK_SIZE
# 绘制方块
draw_block(block_x, block_y, block_shape, block_color)
draw_shadow(shadow_x, shadow_y, shadow_shape, shadow_color)
# 更新游戏状态
clock.tick(10)
if not hit_wall(block_x, block_y + BLOCK_SIZE, block_shape) and not hit_block(block_x, block_y + BLOCK_SIZE, block_shape, block_list):
block_y += BLOCK_SIZE
else:
block_list.append(Block(block_x, block_y, block_type, block_shape, block_color))
clear_block(block_list)
block_type = random.choice(BLOCK_TYPE)
block_color = BLOCK_COLOR[BLOCK_TYPE.index(block_type)]
block_shape = BLOCK_SHAPE[block_type]
block_x = BLOCK_X
block_y = BLOCK_Y
shadow_type = block_type
shadow_color = (100, 100, 100)
shadow_shape = block_shape
shadow_x = block_x
shadow_y = block_y
# 更新屏幕
pygame.display.update()
# 退出pygame
pygame.quit()
```
请注意,这只是一个简单的实现,如果想要改进它,还有很多地方可以改善,例如添加分数和等级系统,增加音效和音乐等等。
阅读全文
相关推荐
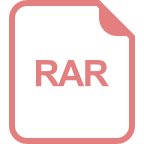
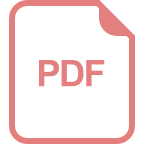













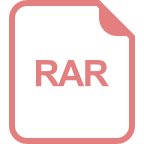