帮我用python写一个俄罗斯方块
时间: 2023-12-31 21:05:27 浏览: 24
作为一个AI助手,我可以为您提供以下Python代码,这是一个简单的俄罗斯方块游戏实现。这个代码需要您自己运行在Python环境中。
```python
import pygame
import random
# 初始化游戏
pygame.init()
# 设置游戏屏幕大小
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
# 设置游戏标题
pygame.display.set_caption("俄罗斯方块")
# 定义颜色
black = (0, 0, 0)
white = (255, 255, 255)
red = (255, 0, 0)
green = (0, 255, 0)
blue = (0, 0, 255)
# 定义方块大小
block_size = 20
# 加载字体
font = pygame.font.SysFont(None, 25)
# 定义方块类
class Block:
def __init__(self):
self.x = screen_width // 2
self.y = 0
self.color = random.choice([red, green, blue])
self.rotation = 0
self.shape = random.choice(["I", "J", "L", "O", "S", "T", "Z"])
self.blocks = {
"I": [(0, -1), (0, 0), (0, 1), (0, 2)],
"J": [(0, -1), (0, 0), (0, 1), (-1, 1)],
"L": [(0, -1), (0, 0), (0, 1), (1, 1)],
"O": [(0, 0), (0, 1), (1, 0), (1, 1)],
"S": [(0, 0), (0, 1), (-1, 0), (1, 1)],
"T": [(0, -1), (0, 0), (0, 1), (1, 0)],
"Z": [(0, 0), (0, 1), (1, 0), (-1, 1)]
}
self.current_blocks = [(self.x, self.y)]
for block in self.blocks[self.shape]:
pos = (self.x + block[0] * block_size, self.y + block[1] * block_size)
self.current_blocks.append(pos)
def move(self, x, y):
self.x += x
self.y += y
self.current_blocks = [(self.x, self.y)]
for block in self.blocks[self.shape]:
pos = (self.x + block[0] * block_size, self.y + block[1] * block_size)
self.current_blocks.append(pos)
def rotate(self):
self.rotation = (self.rotation + 1) % 4
self.current_blocks = [(self.x, self.y)]
for block in self.blocks[self.shape]:
if self.rotation == 0:
pos = (self.x + block[0] * block_size, self.y + block[1] * block_size)
elif self.rotation == 1:
pos = (self.x + block[1] * block_size, self.y - block[0] * block_size)
elif self.rotation == 2:
pos = (self.x - block[0] * block_size, self.y - block[1] * block_size)
elif self.rotation == 3:
pos = (self.x - block[1] * block_size, self.y + block[0] * block_size)
self.current_blocks.append(pos)
def draw(self):
for block in self.current_blocks:
pygame.draw.rect(screen, self.color, (block[0], block[1], block_size, block_size), 0)
# 定义游戏类
class Game:
def __init__(self):
self.score = 0
self.block = Block()
self.game_over = False
self.blocks = []
def add_block(self, block):
for b in block.current_blocks:
self.blocks.append(b)
def remove_line(self):
ys = []
for block in self.blocks:
if block[1] not in ys:
ys.append(block[1])
ys.sort()
for y in ys:
line_blocks = []
for block in self.blocks:
if block[1] == y:
line_blocks.append(block)
if len(line_blocks) == screen_width // block_size:
for block in line_blocks:
self.blocks.remove(block)
for block in self.blocks:
if block[1] < y:
self.blocks.remove(block)
self.blocks.append((block[0], block[1] + block_size))
self.score += 10
def draw(self):
screen.fill(white)
for block in self.blocks:
pygame.draw.rect(screen, black, (block[0], block[1], block_size, block_size), 0)
self.block.draw()
text = font.render("得分:" + str(self.score), True, black)
screen.blit(text, (10, 10))
pygame.display.update()
def check_game_over(self):
for block in self.blocks:
if block[1] == 0:
self.game_over = True
def run(self):
clock = pygame.time.Clock()
while not self.game_over:
for event in pygame.event.get():
if event.type == pygame.QUIT:
self.game_over = True
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
self.block.move(-block_size, 0)
if event.key == pygame.K_RIGHT:
self.block.move(block_size, 0)
if event.key == pygame.K_DOWN:
self.block.move(0, block_size)
if event.key == pygame.K_UP:
self.block.rotate()
if self.block.y < screen_height - block_size * 2:
self.block.move(0, block_size)
else:
self.add_block(self.block)
self.block = Block()
self.remove_line()
self.check_game_over()
self.draw()
clock.tick(5)
text = font.render("游戏结束,得分:" + str(self.score), True, black)
screen.blit(text, (screen_width // 2 - 100, screen_height // 2 - 20))
pygame.display.update()
pygame.time.delay(2000)
# 运行游戏
game = Game()
game.run()
# 退出游戏
pygame.quit()
```
相关推荐
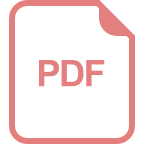
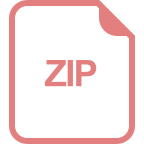
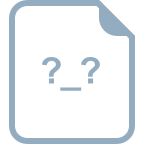









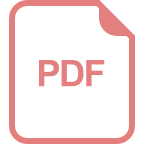
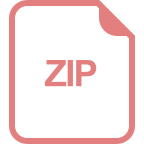
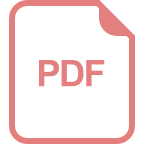
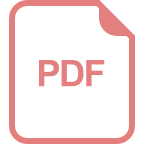