队列示例代码先进先出 C语言
时间: 2023-07-10 14:27:55 浏览: 57
下面是一个简单的队列示例代码,使用C语言实现先进先出:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_QUEUE_SIZE 10
// 定义队列结构体
typedef struct {
int front; // 队首指针
int rear; // 队尾指针
int data[MAX_QUEUE_SIZE]; // 队列元素数组
} Queue;
// 初始化队列
void initQueue(Queue *q) {
q->front = q->rear = 0;
}
// 判断队列是否为空
int isQueueEmpty(Queue *q) {
return q->front == q->rear;
}
// 判断队列是否已满
int isQueueFull(Queue *q) {
return (q->rear + 1) % MAX_QUEUE_SIZE == q->front;
}
// 入队操作
int enqueue(Queue *q, int element) {
if (isQueueFull(q)) {
printf("Queue is full!\n");
return 0;
}
q->data[q->rear] = element;
q->rear = (q->rear + 1) % MAX_QUEUE_SIZE;
return 1;
}
// 出队操作
int dequeue(Queue *q, int *element) {
if (isQueueEmpty(q)) {
printf("Queue is empty!\n");
return 0;
}
*element = q->data[q->front];
q->front = (q->front + 1) % MAX_QUEUE_SIZE;
return 1;
}
int main() {
Queue q;
initQueue(&q);
// 入队操作
int i;
for (i = 0; i < MAX_QUEUE_SIZE; i++) {
enqueue(&q, i);
}
// 出队操作
int element;
while (!isQueueEmpty(&q)) {
dequeue(&q, &element);
printf("%d ", element);
}
printf("\n");
return 0;
}
```
以上代码实现了一个简单的循环队列,队列的长度为10,可以自行修改`MAX_QUEUE_SIZE`的值进行测试。
相关推荐
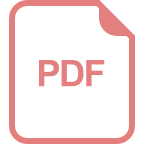
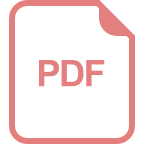
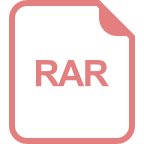














