c语言实现环形队列代码
时间: 2024-06-23 15:00:55 浏览: 233
在C语言中,实现环形队列(Circular Queue)需要使用数组来模拟队列的“先进先出”(FIFO)特性,因为数组的索引可以循环,所以非常适合用来创建环形队列。以下是一个简单的C语言实现环形队列的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100 // 环形队列的最大容量
typedef struct {
int *queue; // 队列元素的存储数组
int front; // 队头指针
int rear; // 队尾指针
} Queue;
// 初始化环形队列
Queue* initQueue() {
Queue *q = (Queue*)malloc(sizeof(Queue));
q->queue = (int*)malloc(MAX_SIZE * sizeof(int));
q->front = q->rear = -1;
return q;
}
// 判断队列是否为空
int isQueueEmpty(Queue *q) {
return q->front == -1;
}
// 判断队列是否已满
int isQueueFull(Queue *q) {
return (q->front + 1) % MAX_SIZE == q->rear;
}
// 入队操作
void enqueue(Queue *q, int value) {
if (isQueueFull(q)) {
printf("Queue is full.\n");
return;
}
q->rear = (q->rear + 1) % MAX_SIZE;
q->queue[q->rear] = value;
if (q->front == -1) {
q->front = 0;
}
}
// 出队操作
int dequeue(Queue *q) {
if (isQueueEmpty(q)) {
printf("Queue is empty.\n");
return -1;
}
int value = q->queue[q->front];
q->front = (q->front + 1) % MAX_SIZE;
if (q->front == q->rear) {
free(q->queue);
free(q);
return value;
}
return value;
}
// 打印队列内容
void printQueue(Queue *q) {
if (isQueueEmpty(q)) {
printf("Queue is empty.\n");
} else {
int index = q->front;
while (index != q->rear) {
printf("%d ", q->queue[index]);
index = (index + 1) % MAX_SIZE;
}
printf("%d\n", q->queue[q->rear]);
}
}
// 示例
int main() {
Queue *q = initQueue();
enqueue(q, 1);
enqueue(q, 2);
enqueue(q, 3);
printQueue(q); // 输出: 1 2 3
dequeue(q);
printQueue(q); // 输出: 2 3
return 0;
}
```
阅读全文
相关推荐
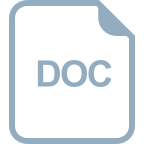
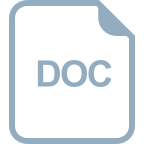


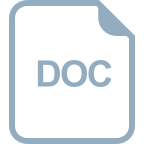



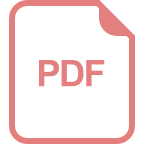
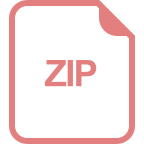
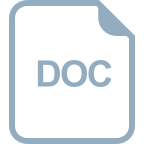
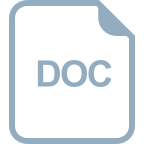



