生成C语言实现使用环形队列实现生产者和消费者的同步与互斥问题的代码
时间: 2024-05-01 22:17:32 浏览: 128
好的,以下是使用环形队列实现生产者和消费者的同步与互斥问题的代码:
```C
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define BUFFER_SIZE 10
#define MAX_ITEM 100
typedef struct {
int buffer[BUFFER_SIZE];
pthread_mutex_t lock;
int readpos, writepos;
pthread_cond_t notempty;
pthread_cond_t notfull;
} queue;
queue *q;
void queue_init() {
q = (queue *)malloc(sizeof(queue));
pthread_mutex_init(&q->lock, NULL);
pthread_cond_init(&q->notempty, NULL);
pthread_cond_init(&q->notfull, NULL);
q->readpos = 0;
q->writepos = 0;
}
void queue_push(int data) {
pthread_mutex_lock(&q->lock);
while ((q->writepos + 1) % BUFFER_SIZE == q->readpos) {
pthread_cond_wait(&q->notfull, &q->lock);
}
q->buffer[q->writepos] = data;
q->writepos++;
if (q->writepos >= BUFFER_SIZE)
q->writepos = 0;
pthread_cond_signal(&q->notempty);
pthread_mutex_unlock(&q->lock);
}
int queue_pop() {
int data;
pthread_mutex_lock(&q->lock);
while (q->writepos == q->readpos) {
pthread_cond_wait(&q->notempty, &q->lock);
}
data = q->buffer[q->readpos];
q->readpos++;
if (q->readpos >= BUFFER_SIZE)
q->readpos = 0;
pthread_cond_signal(&q->notfull);
pthread_mutex_unlock(&q->lock);
return data;
}
void *producer(void *arg) {
int i;
for (i = 0; i < MAX_ITEM; i++) {
queue_push(rand() % 100);
printf("Producer %d produced %d\n", *(int *)arg, q->buffer[q->writepos-1]);
}
return NULL;
}
void *consumer(void *arg) {
int i, data;
for (i = 0; i < MAX_ITEM; i++) {
data = queue_pop();
printf("Consumer %d consumed %d\n", *(int *)arg, data);
}
return NULL;
}
int main() {
int i;
pthread_t producer_threads[2], consumer_threads[2];
queue_init();
int pids[] = {1, 2};
for (i = 0; i < 2; i++) {
pthread_create(&producer_threads[i], NULL, producer, (void *)&pids[i]);
pthread_create(&consumer_threads[i], NULL, consumer, (void *)&pids[i]);
}
for (i = 0; i < 2; i++) {
pthread_join(producer_threads[i], NULL);
pthread_join(consumer_threads[i], NULL);
}
return 0;
}
```
说明:
* 队列使用的是数组实现的环形队列。
* 生产者和消费者共用一个队列,通过互斥锁(`pthread_mutex_t`)实现同步和互斥。
* 生产者和消费者在队列满或空时分别等待和唤醒,使用条件变量(`pthread_cond_t`)实现。
* 启动2个生产者和2个消费者,每个生产者/消费者生产/消费100个数。
阅读全文
相关推荐
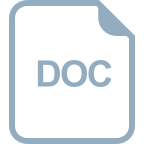
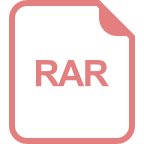
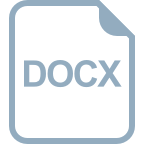















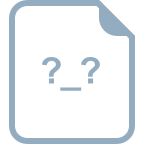