使用c语言实现环形队列
时间: 2024-02-21 19:25:55 浏览: 32
首先,需要定义一个结构体来表示环形队列。
```c
#define QUEUE_SIZE 10
typedef struct {
int front; // 队头指针
int rear; // 队尾指针
int size; // 队列大小
int data[QUEUE_SIZE]; // 数据存储
} CircularQueue;
```
其中,`front` 表示队头指针,`rear` 表示队尾指针,`size` 表示队列大小,`data` 数组用于存储数据。
接下来,需要实现队列的初始化函数。
```c
void initQueue(CircularQueue *queue) {
queue->front = 0;
queue->rear = 0;
queue->size = 0;
}
```
初始化时,将队头指针、队尾指针和队列大小都设置为0。
然后,需要实现入队函数。
```c
int enqueue(CircularQueue *queue, int value) {
if (queue->size == QUEUE_SIZE) {
return -1; // 队列已满
}
queue->data[queue->rear] = value;
queue->rear = (queue->rear + 1) % QUEUE_SIZE;
queue->size++;
return 0;
}
```
如果队列已满,返回-1;否则将数据存储到队尾指针所指向的位置,然后将队尾指针加1,再对队尾指针进行取模操作,以实现环形队列的效果。最后,将队列大小加1,并返回0。
最后,需要实现出队函数。
```c
int dequeue(CircularQueue *queue, int *value) {
if (queue->size == 0) {
return -1; // 队列为空
}
*value = queue->data[queue->front];
queue->front = (queue->front + 1) % QUEUE_SIZE;
queue->size--;
return 0;
}
```
如果队列为空,返回-1;否则将队头指针所指向的数据存储到 `value` 变量中,然后将队头指针加1,再对队头指针进行取模操作,以实现环形队列的效果。最后,将队列大小减1,并返回0。
完整的代码如下:
```c
#include <stdio.h>
#define QUEUE_SIZE 10
typedef struct {
int front; // 队头指针
int rear; // 队尾指针
int size; // 队列大小
int data[QUEUE_SIZE]; // 数据存储
} CircularQueue;
void initQueue(CircularQueue *queue) {
queue->front = 0;
queue->rear = 0;
queue->size = 0;
}
int enqueue(CircularQueue *queue, int value) {
if (queue->size == QUEUE_SIZE) {
return -1; // 队列已满
}
queue->data[queue->rear] = value;
queue->rear = (queue->rear + 1) % QUEUE_SIZE;
queue->size++;
return 0;
}
int dequeue(CircularQueue *queue, int *value) {
if (queue->size == 0) {
return -1; // 队列为空
}
*value = queue->data[queue->front];
queue->front = (queue->front + 1) % QUEUE_SIZE;
queue->size--;
return 0;
}
int main() {
CircularQueue queue;
int value;
initQueue(&queue);
enqueue(&queue, 1);
enqueue(&queue, 2);
enqueue(&queue, 3);
while (dequeue(&queue, &value) == 0) {
printf("%d ", value);
}
printf("\n");
return 0;
}
```
输出:
```
1 2 3
```
相关推荐
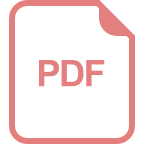
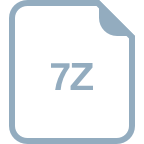
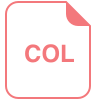
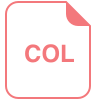
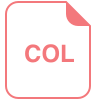











