pythonpygame制作圣诞树
时间: 2024-11-15 07:34:22 浏览: 39
在Python中,通过Pygame库可以轻松地创建一个简单的圣诞节主题游戏或装饰物,如圣诞树。以下是一个基本步骤:
1. **安装Pygame**:首先确保你已经安装了Pygame,如果没有,可以使用pip命令安装:`pip install pygame`
2. **导入所需模块**:开始程序时,需要导入Pygame的基本模块以及用于图形绘制的一些额外模块,如颜色、图像处理等。
```python
import pygame
from pygame.locals import *
```
3. **设置窗口**:初始化Pygame并创建一个新的窗口,设置合适的大小,通常会添加红色背景以模拟节日气氛。
```python
pygame.init()
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Christmas Tree")
background_color = (255, 0, 0)
```
4. **设计圣诞树形状**:可以使用pygame.draw函数画出一个三角形作为树冠,加上装饰如星星、球体等。你可以利用循环和随机位置生成这些元素。
```python
tree_top = (400, 100)
star_colors = [(255, 255, 255), (255, 192, 192), (255, 128, 128)]
stars = [(random.randint(0, screen.get_width()), random.randint(0, screen.get_height())) for _ in range(5)]
for star_pos in stars:
pygame.draw.circle(screen, star_colors[random.randint(0, len(star_colors) - 1)], star_pos, 5)
```
5. **主循环**:在while循环中更新屏幕,检测用户交互,然后关闭窗口。
```python
running = True
while running:
for event in pygame.event.get():
if event.type == QUIT:
running = False
screen.fill(background_color)
pygame.draw.polygon(screen, (0, 255, 0), [(400, 50), (350, 200), (450, 200)])
# 显示圣诞树和星星
screen.blit(pygame.transform.scale(pygame.image.load('tree_image.png'), (300, 400)), tree_top)
pygame.display.flip()
pygame.quit()
```
**注意**: 上述代码示例假设你已经有了一个名为"tree_image.png"的圣诞树图片文件,并已包含在项目中。此外,为了得到更好的视觉效果,你可能需要调整坐标、颜色和大小。
阅读全文
相关推荐
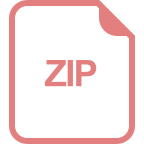
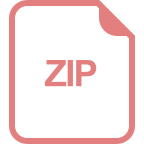
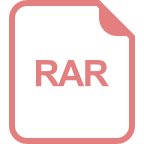

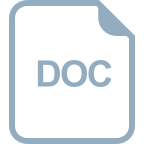

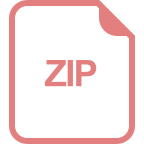
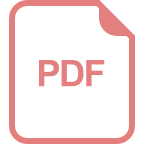
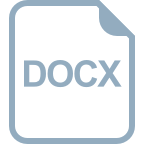
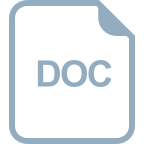
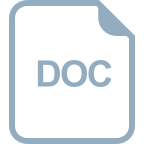
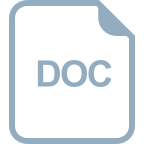
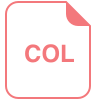
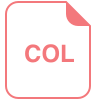
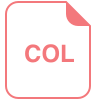



