macos 如何利用hidapi 实现在qt场景画板中二十点划线,详细代码介绍
时间: 2023-11-28 14:47:12 浏览: 75
hidapi 是一个跨平台的库,可以用来访问 USB 和 HID 设备。在 macOS 中,你可以使用 hidapi 库来访问 USB 和 HID 设备,例如数字板和绘图板。
在 Qt 场景画板中二十点划线,你可以使用 hidapi 库来读取数字板或绘图板的坐标数据,然后在 Qt 场景画板中绘制相应的线条。
下面是一个简单的示例代码:
```cpp
#include <QtCore>
#include <QtGui>
#include <QtWidgets>
#include <hidapi/hidapi.h>
class DrawingScene : public QGraphicsScene
{
public:
DrawingScene(QObject *parent = nullptr) : QGraphicsScene(parent)
{
m_pen.setColor(Qt::black);
m_pen.setWidth(2);
}
void mousePressEvent(QGraphicsSceneMouseEvent *event)
{
if (event->button() == Qt::LeftButton) {
m_path.moveTo(event->scenePos());
m_isDrawing = true;
}
}
void mouseMoveEvent(QGraphicsSceneMouseEvent *event)
{
if (m_isDrawing) {
m_path.lineTo(event->scenePos());
addPath(m_path, m_pen);
m_path = QPainterPath(event->scenePos());
}
}
void mouseReleaseEvent(QGraphicsSceneMouseEvent *event)
{
if (event->button() == Qt::LeftButton) {
m_isDrawing = false;
}
}
private:
QPen m_pen;
QPainterPath m_path;
bool m_isDrawing = false;
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
// Create the QGraphicsView and DrawingScene
QGraphicsView view;
DrawingScene scene;
view.setScene(&scene);
view.setWindowTitle("Qt Drawing Board");
view.show();
// Open the HID device
hid_device *dev = hid_open(0x1234, 0x5678, nullptr);
if (!dev) {
qWarning() << "Failed to open HID device";
return -1;
}
// Read the HID report data
unsigned char buf[64];
int res;
while ((res = hid_read(dev, buf, sizeof(buf))) > 0) {
// Parse the HID report data (assuming 20 points)
for (int i = 0; i < 20; i++) {
int x = buf[i * 4] | (buf[i * 4 + 1] << 8);
int y = buf[i * 4 + 2] | (buf[i * 4 + 3] << 8);
QPointF point(x, y);
scene.addEllipse(point, 5, 5, QPen(), QBrush(Qt::red));
}
}
// Close the HID device
hid_close(dev);
return app.exec();
}
```
在上述示例代码中,我们创建了一个 `DrawingScene` 类,该类继承自 `QGraphicsScene` 类,并实现了鼠标事件处理函数,用于绘制线条。然后,我们创建了一个 `QGraphicsView` 对象,将 `DrawingScene` 对象设置为场景,并显示该视图。
接下来,我们使用 `hid_open` 函数打开 HID 设备,并使用 `hid_read` 函数读取 HID 报告数据。假设该报告数据包含 20 个点的坐标信息,我们使用 `buf[i * 4] | (buf[i * 4 + 1] << 8)` 和 `buf[i * 4 + 2] | (buf[i * 4 + 3] << 8)` 来解析每个点的 x 和 y 坐标值,并将其添加到 `DrawingScene` 中。
最后,我们使用 `hid_close` 函数关闭 HID 设备。
请注意,上述示例代码仅用于演示如何使用 hidapi 库读取 HID 设备的数据,并不是完整的实现。你需要根据具体的数字板或绘图板的协议和数据格式来修改代码。
阅读全文
相关推荐


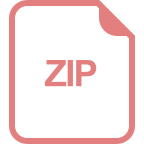
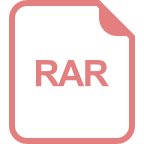
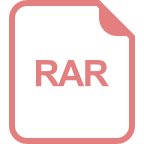
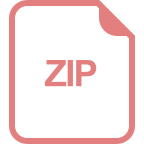
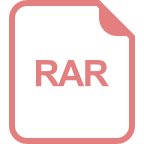
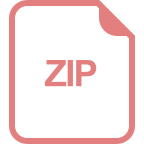
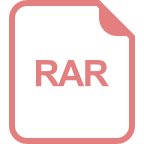
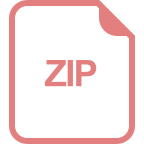
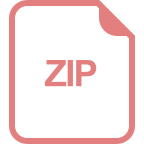
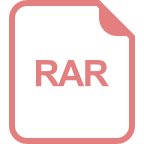
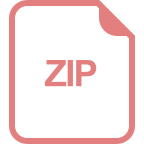
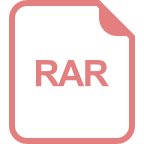
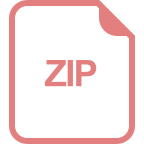
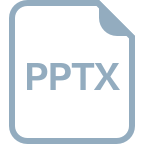