Retinex代码python
时间: 2023-07-02 13:05:25 浏览: 208
Retinex算法是一种用于增强图像亮度和对比度的算法,下面是一个简单的Python实现:
```python
import cv2
import numpy as np
def singleScaleRetinex(img, sigma):
retinex = np.log10(img) - np.log10(cv2.GaussianBlur(img, (0, 0), sigma))
return retinex
def multiScaleRetinex(img, sigma_list):
retinex = np.zeros_like(img)
for sigma in sigma_list:
retinex += singleScaleRetinex(img, sigma)
retinex = retinex / len(sigma_list)
return retinex
def colorRestoration(img, alpha, beta):
img_sum = np.sum(img, axis=2, keepdims=True)
color_restoration = beta * (np.log10(alpha * img) - np.log10(img_sum))
return color_restoration
def automatedMSRCR(img, sigma_list=[15, 80, 250], alpha=125, beta=46):
img = np.float64(img) + 1.0
img_retinex = multiScaleRetinex(img, sigma_list)
img_color = colorRestoration(img, alpha, beta)
img_msrcr = np.multiply(img_retinex, img_color)
img_msrcr = np.uint8(np.minimum(np.maximum(img_msrcr, 0), 255))
return img_msrcr
```
这个实现包含了单尺度Retinex、多尺度Retinex和颜色恢复三个步骤。你可以使用`automatedMSRCR`方法对输入图像进行Retinex增强处理。例如:
```python
img = cv2.imread('input.jpg')
result = automatedMSRCR(img)
cv2.imwrite('output.jpg', result)
```
其中,`sigma_list`是用于多尺度Retinex的高斯核大小列表,`alpha`和`beta`是用于颜色恢复的两个参数,可以根据实际情况进行调整。
阅读全文
相关推荐

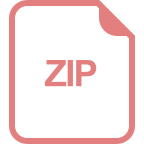





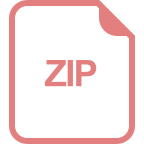







