进程管理实验c语言代码
时间: 2024-12-11 07:15:34 浏览: 12
在C语言中,进行进程管理通常涉及到操作系统级别的编程,例如使用系统调用来创建、控制和终止进程。这里是一个简单的示例,展示了如何使用POSIX API(如fork()和waitpid())来创建一个子进程:
```c
#include <stdio.h>
#include <unistd.h>
#include <sys/wait.h>
#define MAX_CHILDREN 5
int main(int argc, char *argv[]) {
pid_t pid; // 子进程ID
int i;
printf("Parent process started.\n");
for (i = 0; i < MAX_CHILDREN; i++) {
pid = fork(); // 创建子进程
if (pid == 0) { // 子进程
printf("Child %d spawned by parent %d\n", getpid(), getppid());
exit(0); // 子进程结束
} else if (pid > 0) { // 父进程
printf("Parent %d created child %d\n", getpid(), pid);
wait(NULL); // 等待子进程结束
} else { // fork失败
perror("Fork failed");
return -1;
}
}
printf("All children terminated.\n");
return 0;
}
```
在这个例子中,`fork()`函数用于创建新进程,返回值0表示当前进程是新创建的子进程,非零值表示原进程。父进程通过`waitpid()`等待子进程结束。
请注意这只是一个基础示例,实际的进程管理可能会更复杂,涉及到信号处理、资源管理和错误检查等。
阅读全文
相关推荐






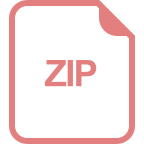










