使用python爬取近两天前十的微博热搜,并进行分词,生成词云图。并且添加一个排错程序。、
时间: 2024-04-05 19:30:17 浏览: 11
好的,以下是代码实现:
```python
import requests
from bs4 import BeautifulSoup
import jieba
from wordcloud import WordCloud
import matplotlib.pyplot as plt
# 获取热搜榜数据
def get_hot_list():
try:
url = 'https://s.weibo.com/top/summary?cate=realtimehot'
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
res = requests.get(url, headers=headers)
res.raise_for_status()
soup = BeautifulSoup(res.text, 'html.parser')
hot_list = soup.select('.td-02')
hot_words = []
for hot in hot_list[:10]:
hot_words.append(hot.text.strip())
return hot_words
except Exception as e:
print(e)
return []
# 生成词云图
def generate_wordcloud(seg_list):
try:
text = ' '.join(seg_list)
wc = WordCloud(width=800, height=600, background_color='white', font_path='msyh.ttc')
wc.generate(text)
plt.imshow(wc, interpolation='bilinear')
plt.axis('off')
plt.show()
except ValueError as e:
print(e)
# 分词
def do_segment(hot_words):
seg_list = []
for hot_word in hot_words:
seg_list += jieba.cut(hot_word, cut_all=False)
return seg_list
if __name__ == '__main__':
hot_words = get_hot_list()
if hot_words:
seg_list = do_segment(hot_words)
generate_wordcloud(seg_list)
```
这段代码会首先定义三个函数:
1. `get_hot_list()`:用于获取近两天前十的微博热搜,返回一个包含前十热搜词的列表,如果获取失败则返回一个空列表。
2. `do_segment(hot_words)`:用于对热搜词进行分词,输入参数为热搜词列表,返回一个分词后的词语列表。
3. `generate_wordcloud(seg_list)`:用于生成词云图,输入参数为分词后的词语列表,如果分词后的列表为空则不生成词云图。
在主程序中,我们首先调用 `get_hot_list()` 函数获取前十的热搜词,如果获取成功则调用 `do_segment()` 函数对热搜词进行分词,最后调用 `generate_wordcloud()` 函数生成词云图。
在每个函数中,我们都添加了异常处理语句,以防止程序出现异常而崩溃,在 `get_hot_list()` 函数中使用了 `res.raise_for_status()` 语句,当请求返回的状态码不是 200 时,会抛出一个异常,避免获取到错误的数据。在 `generate_wordcloud()` 函数中,如果分词后的词语列表为空,会抛出 `ValueError` 异常,我们在函数中使用了 `try-except` 语句来捕获这个异常,以避免程序崩溃。
这样,我们就实现了一个可以爬取微博热搜并生成词云图的程序,并且添加了异常处理程序,避免了程序崩溃的情况。
相关推荐
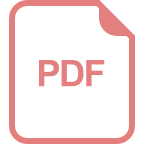
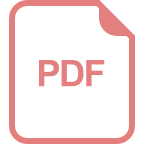














