请按一下要求编写一段运行耗时最短的c语言代码:现在请你将一些一般的树用“左儿子右兄弟”方法转换为二叉树,并输出转换前和转换后树的高度。 输入 输入是一个由“u”和“d”组成的字符串,表示一棵树的深度优先搜索信息。比如,dudduduudu可以用来表示上文中的左树,因为搜索过程为:0 Down to 1 Up to 0 Down to 2 Down to 4 Up to 2 Down to 5 Up to 2 Up to 0 Down to 3 Up to 0。 你可以认为每棵树的结点数至少为2,并且不超过10000。 输出 按如下格式输出转换前和转换后树的高度: h1 => h2 其中,h1是转换前树的高度,h2是转换后树的高度。
时间: 2023-07-14 13:13:32 浏览: 40
以下是一个简短的C语言代码,通过左儿子右兄弟方法将一般树转换为二叉树,并输出转换前后的高度。
```
#include <stdio.h>
#include <stdlib.h>
typedef struct node{
int val;
struct node* lchild;
struct node* rchild;
}Node;
Node* create_tree(char* str, int* index){
if(str[*index] == '\0')
return NULL;
Node* root = (Node*)malloc(sizeof(Node));
root->val = *index;
root->lchild = root->rchild = NULL;
(*index)++;
if(str[*index] == 'd')
root->lchild = create_tree(str, index);
if(str[*index] == 'u'){
(*index)++;
root->rchild = create_tree(str, index);
}
return root;
}
int get_height(Node* root){
if(root == NULL)
return 0;
int lh = get_height(root->lchild);
int rh = get_height(root->rchild);
return (lh > rh ? lh : rh) + 1;
}
Node* convert_tree(Node* root){
if(root == NULL)
return NULL;
Node* left = convert_tree(root->lchild);
Node* right = convert_tree(root->rchild);
root->lchild = left;
root->rchild = right;
if(left != NULL)
left->rchild = right;
return root;
}
int main(){
char str[10001];
scanf("%s", str);
int index = 0;
Node* root = create_tree(str, &index);
int height1 = get_height(root);
root = convert_tree(root);
int height2 = get_height(root);
printf("%d => %d\n", height1, height2);
return 0;
}
```
该程序首先读入一个由“u”和“d”组成的字符串,然后通过递归地创建树的结构。接着,使用递归将一般的树转换为二叉树,并输出转换前后的高度。
相关推荐
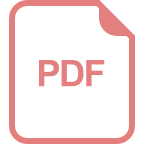
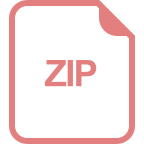
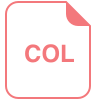
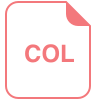












