rrt_connect算法怎么理解
时间: 2024-01-31 19:04:47 浏览: 28
RRT-Connect算法是一种基于Rapidly-exploring Random Tree (RRT)的路径规划算法。它是用来解决在高维、复杂环境中的机器人运动规划问题的。
算法步骤如下:
1. 初始化RRT的起点和终点,并将起点和终点分别作为两棵树的根节点。
2. 在自由空间内随机采样一个点,将该点插入到起点树或终点树中,使得两棵树之间的距离尽可能小。
3. 通过线性插值或其他插值方法,生成一条连接两个树的路径,并检查该路径是否与障碍物相交。如果路径不相交,则将其加入RRT中,并更新树的节点和边。
4. 如果路径相交,则继续随机采样新节点,并尝试连接两棵树,直到找到一条不相交的路径或者达到最大迭代次数。
5. 返回最优路径(即连接两棵树的路径中长度最小的那条路径)。
RRT-Connect算法的优点是可以快速地在高维空间中找到一条可行路径,并且可以在不同的环境中使用。缺点是可能会出现局部最优解,因此需要进行多次随机采样和路径连接。
相关问题
rrt_connect算法c++实现
以下是RRT-Connect算法的C++实现示例。
```cpp
#include <iostream>
#include <cmath>
#include <vector>
#include <cstdlib>
#include <ctime>
#include <chrono>
using namespace std;
// 定义状态结构体
struct State {
double x;
double y;
};
// 定义节点结构体
struct Node {
State state;
Node* parent;
};
// 定义RRT-Connect类
class RRTConnect {
public:
RRTConnect(State start, State goal, double map_width, double map_height, double step_size);
void planning();
vector<State> getPath();
private:
Node* getRandomNode();
Node* getNearestNode(Node* node);
bool isCollide(State state);
bool isReach(State s1, State s2);
void addVertex(Node* parent, Node* child);
State generateRandomState();
vector<Node*> tree1;
vector<Node*> tree2;
State start_;
State goal_;
double map_width_;
double map_height_;
double step_size_;
double goal_radius_ = 0.5;
int max_iterations_ = 5000;
};
// RRT-Connect算法类构造函数
RRTConnect::RRTConnect(State start, State goal, double map_width, double map_height, double step_size)
: start_(start), goal_(goal), map_width_(map_width), map_height_(map_height), step_size_(step_size) {
Node* start_node = new Node{ start_, nullptr };
Node* goal_node = new Node{ goal_, nullptr };
tree1.push_back(start_node);
tree2.push_back(goal_node);
srand(time(nullptr)); // 初始化随机数种子
}
// 获取随机节点
Node* RRTConnect::getRandomNode() {
double x = (double)rand() / RAND_MAX * map_width_;
double y = (double)rand() / RAND_MAX * map_height_;
return new Node{ State{x, y}, nullptr };
}
// 获取最近节点
Node* RRTConnect::getNearestNode(Node* node) {
Node* nearest_node = tree1.front();
double min_dist = hypot(nearest_node->state.x - node->state.x, nearest_node->state.y - node->state.y);
for (auto n : tree1) {
double dist = hypot(n->state.x - node->state.x, n->state.y - node->state.y);
if (dist < min_dist) {
nearest_node = n;
min_dist = dist;
}
}
return nearest_node;
}
// 判断状态是否碰撞
bool RRTConnect::isCollide(State state) {
double obs_x = map_width_ / 2;
double obs_y = map_height_ / 2;
double obs_r = 2.0;
double dist = hypot(state.x - obs_x, state.y - obs_y);
return dist < obs_r;
}
// 判断两个状态是否可达
bool RRTConnect::isReach(State s1, State s2) {
double dist = hypot(s1.x - s2.x, s1.y - s2.y);
return dist < step_size_;
}
// 添加节点
void RRTConnect::addVertex(Node* parent, Node* child) {
child->parent = parent;
tree1.push_back(child);
}
// 生成随机状态
State RRTConnect::generateRandomState() {
double x = (double)rand() / RAND_MAX * map_width_;
double y = (double)rand() / RAND_MAX * map_height_;
return State{ x, y };
}
// RRT-Connect算法主函数
void RRTConnect::planning() {
for (int i = 0; i < max_iterations_; i++) {
Node* q_rand = getRandomNode();
Node* q_near = getNearestNode(q_rand);
State new_state;
if (isReach(q_near->state, q_rand->state)) {
new_state = q_rand->state;
} else {
double theta = atan2(q_rand->state.y - q_near->state.y, q_rand->state.x - q_near->state.x);
new_state = State{ q_near->state.x + step_size_ * cos(theta), q_near->state.y + step_size_ * sin(theta) };
}
if (isCollide(new_state)) {
continue;
}
Node* new_node = new Node{ new_state, nullptr };
addVertex(q_near, new_node);
if (isReach(new_state, goal_)) {
addVertex(tree2.front(), new Node{ goal_, nullptr });
break;
}
swap(tree1, tree2);
}
}
// 获取路径
vector<State> RRTConnect::getPath() {
vector<State> path;
Node* node = tree1.back();
while (node) {
path.push_back(node->state);
node = node->parent;
}
reverse(path.begin(), path.end());
node = tree2.front()->parent;
while (node) {
path.push_back(node->state);
node = node->parent;
}
return path;
}
int main() {
State start{ 1.0, 1.0 };
State goal{ 9.0, 9.0 };
RRTConnect rrt(start, goal, 10.0, 10.0, 0.5);
auto start_time = chrono::high_resolution_clock::now();
rrt.planning();
auto end_time = chrono::high_resolution_clock::now();
auto duration = chrono::duration_cast<chrono::milliseconds>(end_time - start_time);
cout << "Time: " << duration.count() << "ms" << endl;
vector<State> path = rrt.getPath();
for (auto state : path) {
cout << "(" << state.x << ", " << state.y << ")" << endl;
}
return 0;
}
```
这个示例中,我们使用了两个树来进行RRT-Connect算法的搜索。其中,tree1是从起点出发的树,tree2是从终点出发的树。在每次迭代中,我们随机生成一个节点q_rand,并在tree1中寻找距离q_rand最近的节点q_near。然后,我们通过扩展q_near,生成新的节点new_node,并将其添加到tree1中。如果new_node离终点goal足够近,我们将生成一个新的终点节点,并结束搜索。在添加完new_node后,我们交换tree1和tree2,以便下一次迭代时,tree1成为从终点开始的树,tree2成为从起点开始的树。
值得注意的是,这个示例中我们假设地图中只有一个障碍物,障碍物的位置和大小都是固定的。在实际应用中,我们需要根据实际场景来确定障碍物的位置和大小。
RRT-connect算法
RRT-connect算法是一种用于路径规划的算法,它基于快速随机树(Rapidly-exploring Random Trees, RRT)算法的思想。RRT-connect算法通过构建两棵RRT树来搜索起点到终点的可行路径。
首先,算法会在起点和终点分别构建一棵RRT树。每次迭代,算法会随机生成一个节点,并将该节点连接到最近的树节点。然后,检查连接的路径是否与障碍物相交。如果路径是可行的,则将新节点添加到树中。这个过程会一直重复,直到两棵树连接起来,即从起点到终点存在一条路径。
RRT-connect算法的优点是可以在高维空间中搜索路径,并且在大多数情况下能够找到最优解。它适用于不需要全局最优解的问题,并且对于具有多个自由度的系统,例如机器人运动规划和无人机路径规划等领域,有着广泛的应用。
相关推荐
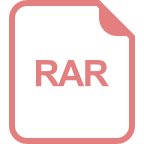












