rrt-connect算法matlab代码
时间: 2023-08-29 18:06:51 浏览: 338
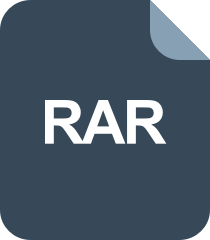
RRTconnect的matlab代码

当然,我可以为你提供 RRT-Connect 算法的 MATLAB 代码示例。RRT-Connect 是一种基于 Rapidly-exploring Random Trees (RRT) 的路径规划算法,用于在两个给定点之间查找可行路径。以下是一个简单的实现:
```matlab
% RRT-Connect Algorithm
function path = rrt_connect(start, goal, obstacles, max_iter, step_size)
% Initialize trees
tree_start = start;
tree_goal = goal;
for i = 1:max_iter
% Randomly sample a point
if rand() < 0.5
rand_point = [rand()*100, rand()*100]; % Modify according to your workspace size
else
rand_point = goal;
end
% Extend tree_start towards rand_point
nearest_start = nearest_neighbor(tree_start, rand_point);
new_point_start = steer(nearest_start, rand_point, step_size);
if ~collision(new_point_start, nearest_start, obstacles)
tree_start = add_node(tree_start, nearest_start, new_point_start);
% Connect tree_goal towards new_point_start
nearest_goal = nearest_neighbor(tree_goal, new_point_start);
new_point_goal = steer(nearest_goal, new_point_start, step_size);
if ~collision(new_point_goal, nearest_goal, obstacles)
tree_goal = add_node(tree_goal, nearest_goal, new_point_goal);
% Check if the trees have met
if norm(new_point_start - new_point_goal) <= step_size
path = generate_path(tree_start, tree_goal);
return;
end
end
end
% Swap trees if necessary
if rand() < 0.5
temp = tree_start;
tree_start = tree_goal;
tree_goal = temp;
end
end
% No path found
error('No feasible path found');
end
% Helper functions
function nearest_node = nearest_neighbor(tree, point)
distances = vecnorm(tree - point, 2, 2);
[~, index] = min(distances);
nearest_node = tree(index, :);
end
function new_point = steer(from, to, step_size)
direction = (to - from) / norm(to - from);
new_point = from + direction * step_size;
end
function is_collision = collision(point, from, obstacles)
for i = 1:size(obstacles, 1)
if norm(point - from) <= norm(obstacles(i, :) - from)
is_collision = true;
return;
end
end
is_collision = false;
end
function new_tree = add_node(tree, from, to)
new_tree = [tree; to];
end
function path = generate_path(tree_start, tree_goal)
path_start = trace_path(tree_start);
path_goal = flip(trace_path(tree_goal));
path = [path_start; path_goal];
end
function path = trace_path(tree)
path = tree(end, :);
while ~isempty(path) && ~isequal(path(1, :), tree(1, :))
parent_index = find(ismember(tree, path(1, :), 'rows'));
path = [tree(parent_index, :); path];
end
end
```
请注意,这只是一个简单的示例代码,你需要根据你的具体场景进行修改和适配。你需要提供起点、终点、障碍物、最大迭代次数和步长等参数来调用 `rrt_connect` 函数。如果找到可行路径,它将返回一系列点构成的路径。如果没有找到可行路径,它将抛出错误。
希望这可以帮助到你!如果你有任何其他问题,请随时问我。
阅读全文
相关推荐
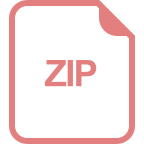
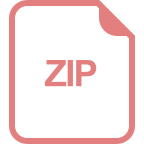
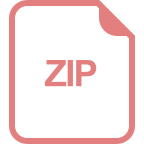
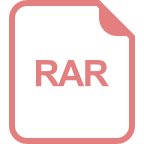
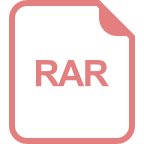
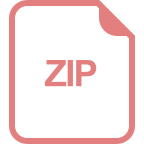
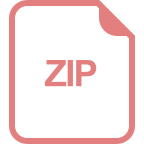
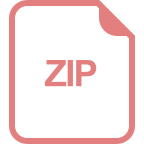
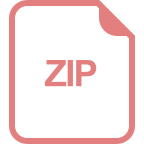





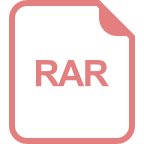
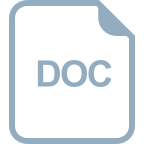