c语言设计一个算法,通过一趟遍历在单链表中确定值最大的结点。
时间: 2023-12-03 07:44:20 浏览: 52
以下是使用C语言实现在单链表中寻找值最大的节点的算法:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
void findMax(struct Node* head) {
int max = head->data;
struct Node* maxNode = head;
while (head != NULL) {
if (head->data > max) {
max = head->data;
maxNode = head;
}
head = head->next;
}
printf("The node with the maximum value is: %d\n", maxNode->data);
}
int main() {
struct Node* head = NULL;
struct Node* second = NULL;
struct Node* third = NULL;
head = (struct Node*)malloc(sizeof(struct Node));
second = (struct Node*)malloc(sizeof(struct Node));
third = (struct Node*)malloc(sizeof(struct Node));
head->data = 1;
head->next = second;
second->data = 5;
second->next = third;
third->data = 3;
third->next = NULL;
findMax(head);
return 0;
}
```
在这个例子中,我们通过定义一个结构体`Node`来表示单链表的节点,每个节点包含有一个整数`data`和一个指向下一个节点的指针`next`。`findMax`函数遍历整个单链表,并在遍历过程中保持跟踪最大值和对应的节点。最后,我们输出找到的值最大的节点的值。
在`main`函数中,我们创建了一个简单的单链表,其中包含三个节点(数据分别为1、5和3),并调用`findMax`函数来查找值最大的节点。
相关推荐
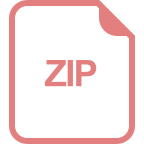
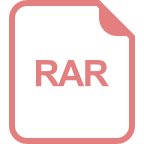














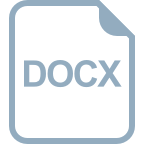