设计一个算法,通过一趟遍历查找单链表L中 值最小的结点,并删除该结点。用c语言
时间: 2024-10-14 21:12:50 浏览: 57
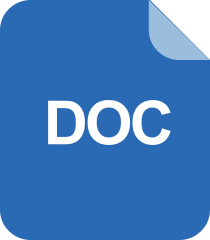
二叉树遍历算法的应用

设计一个C语言算法来找到并删除单链表L中的最小元素可以分为三个步骤:
1. 初始化:创建两个指针`current`和`minNode`,分别指向链表的头节点和假设为最大值的节点。如果链表为空,直接返回。
```c
struct ListNode* findMin(struct ListNode* head) {
if (head == NULL) {
return NULL;
}
struct ListNode* current = head;
struct ListNode* minNode = head;
}
```
2. 遍历链表:在循环中,每次比较当前节点的值和已知最小值,如果当前节点的值更小,则更新`minNode`。
```c
while (current != NULL) {
if (current->val < minNode->val) {
minNode = current;
}
current = current->next;
}
```
3. 删除最小节点:找到最小节点后,需要处理两个情况:
- 如果链表只有一个元素,直接删除并返回NULL;
- 否则,从`minNode`的下一个节点开始连接原来的前驱节点,即`minNode->prev->next = minNode->next`。
```c
if (minNode == head) { // 单个元素的情况
head = head->next;
} else {
minNode->prev->next = minNode->next; // 更新前驱节点的next指针
}
return head; // 返回新的链表头
```
完整函数示例:
```c
struct ListNode* deleteMin(struct ListNode* head) {
if (head == NULL || head->next == NULL) {
return head;
}
struct ListNode* current = head;
struct ListNode* minNode = head;
while (current != NULL && current->next != NULL) {
if (current->val < minNode->val) {
minNode = current;
}
current = current->next;
}
if (current == NULL) { // 最小值就是最后一个节点
head = head->next;
} else {
minNode->prev->next = minNode->next;
}
return head;
}
```
阅读全文
相关推荐
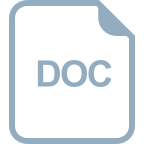
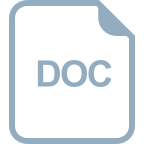















