用C语言结构体变量编写双联动不同角度的步进电机程序
时间: 2023-06-17 15:02:13 浏览: 53
以下是一个使用结构体变量编写的双联动不同角度的步进电机程序的示例。假设我们有两个步进电机,每个步进电机都有4个相位线,分别为A1, A2, B1和B2。我们使用两个结构体变量来存储每个步进电机的状态和角度,结构体变量包括步进电机的4个相位线的状态以及步进电机的当前角度。
```c
#include <stdio.h>
struct StepperMotor {
int A1;
int A2;
int B1;
int B2;
int angle;
};
void step(struct StepperMotor *motor, int direction)
{
// 计算下一个状态
int next_A1 = motor->A2;
int next_A2 = motor->B1;
int next_B1 = motor->A1;
int next_B2 = motor->B2;
// 根据方向调整下一个状态
if (direction == 1) {
motor->angle += 45;
} else {
motor->angle -= 45;
}
// 更新状态
motor->A1 = next_A1;
motor->A2 = next_A2;
motor->B1 = next_B1;
motor->B2 = next_B2;
}
int main()
{
// 初始化电机状态和角度
struct StepperMotor motor1 = {1, 0, 0, 1, 0};
struct StepperMotor motor2 = {1, 1, 0, 0, 0};
// 模拟旋转
for (int i = 0; i < 8; i++) {
printf("Motor1 angle: %d, Motor2 angle: %d\n", motor1.angle, motor2.angle);
if (i % 2 == 0) {
step(&motor1, 1);
step(&motor2, -1);
} else {
step(&motor1, -1);
step(&motor2, 1);
}
}
return 0;
}
```
在这个程序中,我们使用了一个 `step` 函数来模拟步进电机的旋转。该函数接受一个指向 `StepperMotor` 结构体变量的指针以及一个方向参数。该函数首先计算下一个状态,然后根据方向参数调整下一个状态,并更新结构体变量的状态和角度。
在 `main` 函数中,我们初始化了两个 `StepperMotor` 结构体变量,并模拟了它们的旋转。我们交替地调用 `step` 函数来模拟两个电机的旋转,每个电机每次旋转45度。我们使用 `printf` 函数来输出每个电机的角度。
相关推荐
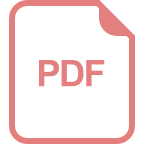
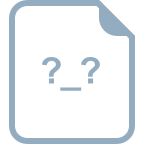














